7 API Interface Function List¶
7.1 Unity related¶
Please find APIs provided by UnityXR plugins at https://docs.unity3d.com/Manual/VRReference.html
Currently supported modules:
XRDisplaySubsystem :https://docs.unity3d.com/Manual/xrsdk-display.html
XRInputSubsystem :https://docs.unity3d.com/Manual/xrsdk-input.html
XRNodeState :https://docs.unity3d.com/ScriptReference/XR.XRNodeState.html
XRSettings:https://docs.unity3d.com/ScriptReference/XR.XRSettings.html
XRStats:https://docs.unity3d.com/ScriptReference/XR.XRStats.html
7.2 Controller related¶
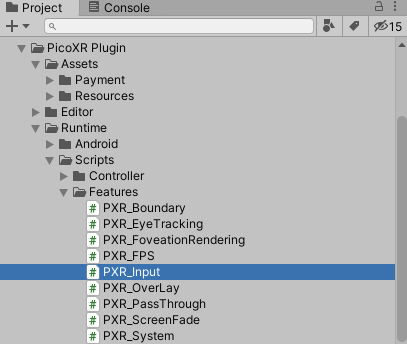
Fig 7.1 File path of PXR_Input.cs
IsControllerConnected¶
Function name: public static bool IsControllerConnected(Controller controller)
Functions: Get the connection status of controller
Parameter: Controller - left and right controllers
Return value: True - connected, false - not connected
Method of calling: PXR_Input.IsControllerConnected (controller)
GetDominantHand¶
Function name: public static Controller GetDominantHand()
Functions: Get the main controller
Return value: Controller.LeftController/Controller.RightController
Method of calling: PXR_Input.GetDominantHand()
SetDominantHand¶
Function name: public static void SetDominantHand(Controller controller)
Functions: Set the main controller
Parameter: Controller - left and right controllers
Return value: None
Method of calling: PXR_Input.SetDominantHand (controller)
SetControllerVibration¶
Function name: public static void SetControllerVibration(float strength, int time, Controller controller)
Functions: Vibrate the controller
Parameter: Strength -vibration strength: 0-1; time of duration (unit: ms); time: 0-65535; controller- left and right controllers
Return value: None
Method of calling: PXR_Input.SetControllerVibration (strength, time, controller)
GetControllerPredictPosition¶
Functions: public static Vector3 UPvr_GetControllerPredictPosition(Controller controller, float predictTime)
Functions: Acquire predicted position of controller
Parameter: controller: controller enum for left and right hand, predictTime: time to predict (in milliseconds)
Return value: Predicted position value
Method of calling: PXR_Input.GetControllerPredictPosition(controller, predictTime)
Notes: Added in version 1.2.2
GetControllerPredictRotation¶
Functions: public static Quaternion GetControllerPredictRotation(Controller controller, float predictTime)
Functions: Acquire predicted rotation of controller
Parameter: controller: controller enum for left and right hand, predictTime: time to predict (in milliseconds)
Return value: Predicted position value
Method of calling: PXR_Input.GetControllerPredictRotation(controller, predictTime)
Notes: Added in version 1.2.2
SetControllerOriginOffset¶
Function name: public static void SetControllerOriginOffset(Controller controller, Vector3 offset)
Functions: Add an offset to controller origin
Parameter: controller: controller enum,offset:positional offset (in meters)
Return value: None
Method of calling: PXR_Input.SetControllerOriginOffset(controller, offset)
Notes: Added in version 1.2.3
GetPredictedDisplayTime¶
Function name: public static float GetPredictedDisplayTime()
Functions: Get predicted display time delay (the interval between time of frame rendering end and time of actual display)
Parameter: None
Return value: Delay time (miliseconds)
Method of calling: PXR_System.GetPredictedDisplayTime()
Notes: Added in version 1.2.3
GetControllerHandness(New)¶
Function name: public static Controller GetControllerHandness ()
Functions: Get the current master control controller index
Parameter: None
Return value: Controller
Method of calling: PXR_Input. GetControllerHandness ();
7.3 Eye tracking related¶
The interfaces in this chapter are applicable for Neo2 Eye and Neo3 Pro Eye.
GetCombineEyeGazePoint¶
Function name: public static bool GetCombineEyeGazePoint(out Vector3 point)
Functions: Get the position of the combined gaze point
Parameter: Receive the vector 3 returned by the result
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking.GetCombineEyeGazePoint ()
GetCombineEyeGazeVector¶
Function name: public static bool GetCombineEyeGazeVector(out Vector3 vector)
Functions: Get the direction of the combined gaze point
Parameter: Receive the vector 3 returned by the result
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking.GetCombineEyeGazeVector(out Vector3 vector)
GetLeftEyeGazePoint¶
Function name: public static bool GetLeftEyeGazePoint (out Vector3 vector)
Functions: Get the origin of left eye gaze point
Parameter: Receive the vector 3 returned by the result
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking . GetLeftEyeGazePoint (out Vector3 vector)
GetRightEyeGazePoint¶
Function name: public static bool GetRightEyeGazePoint (out Vector3 vector)
Functions: Get the origin of left eye gaze point
Parameter: Receive the vector 3 returned by the result
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking . GetRightEyeGazePoint (out Vector3 vector)
GetLeftEyeGazeOpenness¶
Function name: public static bool GetLeftEyeGazeOpenness (out float openness)
Functions: Get the openness/closeness of left eye
Parameter: Receive the float returned by the result; range: 0.0 - 1.0, 0.0 - complete closeness, 1.0 - complete openness
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking . GetLeftEyeGazeOpenness (out float openness)
GetRightEyeGazeOpenness¶
Function name: public static bool GetRightEyeGazeOpenness(out float openness)
Functions: Get the openness/closeness of right eye
Parameter: Receive the float returned by the result; range: 0.0 - 1.0, 0.0 - complete closeness, 1.0 - complete openness
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking . GetRightEyeGazeOpenness (out float openness)
GetLeftEyePoseStatus¶
Function name: public static bool GetLeftEyePoseStatus(out uint status)
Functions: Get the pose status of left eye
Parameter: Receive the int returned by the result; 0- not good, 1-good
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking.GetLeftEyePoseStatus (out uint status)
GetRightEyePoseStatus¶
Function name: public static bool GetRightEyePoseStatus (out uint status)
Functions: Get the pose status of right eye
Parameter: Receive the int returned by the result; 0- not good, 1-good
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking.GetRightEyePoseStatus (out uint status)
GetCombinedEyePoseStatus¶
Function name: public static bool GetCombinedEyePoseStatus(out uint status)
Functions: Get the combined pose status
Parameter: Receive the int returned by the result; 0- not good, 1-good
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking.GetCombinedEyePoseStatus (out uint status)
GetLeftEyePositionGuide¶
Function name: public static bool GetLeftEyePositionGuide (out Vector3 position)
Functions: Get the position guide of left eye
Parameter: Receive the vector 3 returned by the result
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking.GetLeftEyePositionGuide (out Vector3 position)
GetRightEyePositionGuide¶
Function name: public static bool GetRightEyePositionGuide (out Vector3 position)
Functions: Get the position guide of right eye
Parameter: Receive the vector 3 returned by the result
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking.GetRightEyePositionGuide (out Vector3 position)
GetFoveatedGazeDirection¶
Function name: public static bool GetFoveatedGazeDirection(out Vector3 position)
Functions: Get the foveated gaze direction
Parameter: Receive the vector 3 returned by the result
Return value: True - succeed, false - failed
Method of calling: PXR_EyeTracking.GetFoveatedGazeDirection (out Vector3 position)
7.4 Foveated rendering related¶
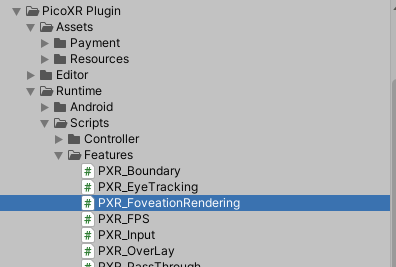
Fig 7.2 File path of PXR_FoveationRendering.cs
GetFoveatedRenderingLevel¶
Function name: public static FoveationLevel GetFoveationLevel()
Functions: Get current foveated rendering level
Parameter: None
Return value: Current foveated rendering level
Method of calling: PXR_FoveationRendering. GetFoveationLevel()
SetFoveatedRenderingLevel¶
Function name: public static void SetFoveationLevel (FoveationLevel level)
Functions: Set foveated rendering level
Parameter: Targeted foveated rendering level with 4 levels: None, Low, Med, and High. None means “disabled”.
Return value: None
Method of calling: PXR_FoveationRendering.SetFoveationLevel(level)
SetFoveatedRenderingParameters¶
Function name: public static void SetFoveationParameters(foveationGainX, foveationGainY, foveationArea, foveationMinimum)
Functions: Set current foveated rendering parameters
Parameter: Target foveated rendering parameters
foveationGainX/Y: Ratio of peripheral pixel scaling down in X/Y direction, larger value indicates more ratio scaling down.
foveationArea: The resolution of the area around the gazing point with the ffrAreaValue as radius will stay the same.
foveationMinimum: Texture coordinate parameter of texture filtering function
SDK provides four levels:
Level | foveationGain | foveationArea | foveationMinimum |
---|---|---|---|
Low | (3.0f,3.0f) | 1.0f | 0.125f |
Med | (4.0f,4.0f) | 1.0f | 0.125f |
High | (6.0f,6.0f) | 1.0f | 0.0625f |
Top High | (7.0f,7.0f) | 0.0f | 0.0625f |
Return value: None
Method of calling: PXR_FoveationRendering. SetFoveatedRenderingParameters(foveationGainX, foveationGainY, foveationArea, foveationMinimum)
7.5 Safety Boundary related¶
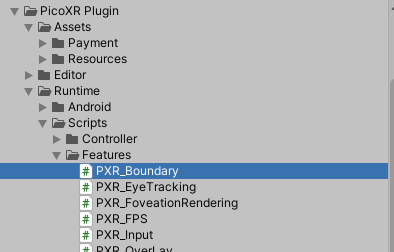
Fig 7.3 File path of PXR_Boundary.cs
GetConfigured¶
Function name: public static bool GetConfigured()
Function: Return result of Safety Boundary configuring
Parameter: None
Return value: true: Success false: Failure
Method of calling: PXR_Boundary.GetConfigured()
GetEnabled¶
Function name: public static bool GetEnabled ()
Functions: Get the enabling status of safety zone protection system
Parameter: None
Return value: True - enabled, false - not enabled
Method of calling: PXR_Boundary.GetEnabled ()
SetVisible¶
Function name: public static void SetVisible(bool value)
Functions: Force to set whether Safety Boundary is visible (Note: Safety Boundary activation and user configuration in system settings will overwrite this interface’s action)
Parameter: value: whether the Safety Boundary is visible or not
Return value: None
Method of calling: PXR_Boundary.SetVisible(value)
GetVisible¶
Function name:public static bool GetVisible()
Functions: Get whether the safety boundary is visible or not
Parameter: None
Return Value:Whether the safety area is visible or not
Method of calling: PXR_Boundary.GetVisible()
TestNode¶
Function name: public static BoundaryTestResult TestNode(BoundaryTrackingNode node, BoundaryType boundaryType)
Functions: Return testing results of tracking nodes to specific boundary types
Parameter: node: tracking node, boundaryType: boundary type
Return Value: BoundaryTestResult which is a struct of boundary test result
public struct BoundaryTestResult
{
public bool IsTriggering; // If boundary is triggered
public float ClosestDistance; // Minimum distance of tracking nodes and boundary
public Vector3 ClosestPoint; // Closest point of tracking nodes and boundary
public Vector3 ClosestPointNormal; // Normal of closest point
}
Method of calling: PXR_Boundary.TestNode(node, boundaryType);
TestPoint¶
Function name:public static BoundaryTestResult TestPoint(Vector3 point, BoundaryType boundaryType)
Functions:Return testing results of a 3-dimensional point coordinate to a specific boundary type
Parameters:point:coordinate of point,boundaryType:type of boundary
Return Value:BoundaryTestResult which is a struct of boundary test result
public struct BoundaryTestResult
{
public bool IsTriggering; // If boundary is triggered
public float ClosestDistance; // Minimum distance of tracking nodes and boundary
public Vector3 ClosestPoint; // Closest point of tracking nodes and boundary
public Vector3 ClosestPointNormal; // Normal of closest point
}
Method of calling: PXR_Boundary .TestPoint(point, boundaryType);
GetGeometry¶
Function name:public static Vector3[]GetGeometry(BoundaryType boundaryType)
Functions:Return the collection of boundary points
Parameter:boundaryType:type of safety boundary
Return Value:Vector3[]: Collection of safety boundary points
Method of Calling: PXR_Boundary .GetGeometry(boundaryType);
GetDimensions¶
Function name: public static Vector3 GetDimensions (BoundaryType boundaryType)
Functions: Get the size of the custom security boundary PlayArea
Parameter: boundaryType- boundary type
Return Value: Vector3, x: long side of PlayArea, y: 1, z: short side of PlayArea; If the current place is within security boundary, the value of V3 is (0, 0, 0)
Method of Calling: PXR_Boundary.GetDimensions (boundaryType);
GetDialogState¶
Function name: public static int GetDialogState ()
Functions: Get the boundary dialog state
Parameter: None
Return Value: NothingDialog = -1,GobackDialog = 0,ToofarDialog = 1,LostDialog = 2,LostNoReason = 3,LostCamera = 4, LostHighLight = 5,LostLowLight = 6,LostLowFeatureCount = 7,LostReLocation = 8
Method of calling: PXR_Boundary.GetDialogState();
7.6 SeeThrough Camera related¶
EnableSeeThroughManual¶
Function name: public static void EnableSeeThroughManual(bool value)
Functions: Get the camera picture of Neo2/Neo3 device and use it as the environmental background
Parameter: value:Open or not SeeThrough,True on, false off
Return Value: None
Method of calling: PXR_Boundary.EnablssSeeThroughManual (value);
Others: 1. The clear flags of the camera is set to solid color 2. The background color of the camera is set to 0 for the alpha channel
PassThroughSystem¶
Functions: Get camera imaging of Neo2/Neo3 devices
Method of calling: Refer to section 8.11 PassThrough
7.7 Device related¶
Note: please call the interface InitAudioDevice to initialize before using services such as power, volume and brightness
InitAudioDevice¶
Function name: public bool InitAudioDevice()
Functions: Initialize the volume device
Parameters: True: success false: failure
Method of calling: PXR_System.InitAudioDevice()
StartAudioReceiver¶
Function name: public bool StartAudioReceiver(string startreceiver)
Functions: Turn on the volume service
Parameters:Gameobject name to turn on the volume
Return value: True: success false: failure
Method of calling: PXR_System.StartAudioReceiver(startreceiver)
setAudio¶
Function name: public void setAudio(string s)
Functions: Callback when volume changes
Parameters: Current volume
Return value: None
Method of calling: You can add logic directly in this method or use delegate to call it
StopAudioReceiver¶
Function name: public bool StopAudioReceiver()
Functions: Turn off the volume service
Parameters: None
Return value: True: success false: failure
Method of calling: PXR_System.StopAudioReceiver()
StartBatteryReceiver¶
Function name: public bool StartBatteryReceiver(string startreceiver)
Functions: Turn on the power service
Parameters: Gameobject name to turn on the power
Return value: True: success false: failure
Method of calling: PXR_System.StartBatteryReceiver(startreceiver)
setBattery¶
Function name: public void setBattery(string s)
Functions: Callback when battery changes
Parameters: Current power (range: 0.00~1.00)
Return value: None
Method of calling: You can add logic directly in this method or use delegate to call it
StopBatteryReceiver¶
Function name: public bool StopBatteryReceiver()
Functions: Turn off the power service
Parameters: None
Return value: True: success false: failure
Method of calling: PXR_System.StopBatteryReceiver()
GetMaxVolumeNumber¶
Function name: public int GetMaxVolumeNumber()
Functions: Get the maximum volume (need to initialize the volume device)
Parameters: None
Return value: Maximum volume
Method of calling: PXR_System.GetMaxVolumeNumber()
GetCurrentVolumeNumber¶
Function name: public int GetCurrentVolumeNumber()
Functions: Get the current volume (need to initialize the volume device)
Parameters: None
Return value: Current volume value (range: 0~15)
Method of calling: PXR_System.GetCurrentVolumeNumber()
VolumeUp¶
Function name: public bool VolumeUp()
Functions: Increase the volume (need to initialize the volume device)
Parameters: None
Return value: True: success false: failure
Method of calling: PXR_System.VolumeUp()
VolumeDown¶
Function name: public bool VolumeDown()
Functions: decrease the volume (need to initialize the volume device)
Parameters: None
Return value: True: success false: failure
Method of calling: PXR_System.VolumeDown()
SetVolumeNum¶
Function name: public bool SetVolumeNum(int volume)
Functions: Set the volume (need to initialize the volume device)
Parameters: Set the volume value (the range of volume value is 0~15)
Return value: True: success false: failure
Method of calling: PXR_System.SetVolumeNum(volume)
GetCommonBrightness¶
Function name: public int GetCommonBrightness()
Functions: Acquire that brightness value of the current general equipment
Parameters: None
Return value: Current brightness value (the range of brightness value is 0~255)
Method of calling: PXR_System.GetCommonBrightness()
SetCommonBrightness¶
Function name: public bool SetCommonBrightness(int brightness)
Functions: Set brightness value of current general equipment
Parameters: Brightness value (the range of brightness value is 0~255)
Return value: True: success, false: failure
Method of calling: PXR_System.SetCommonBrightness(brightness)
SetExtraLatencyMode¶
Function name: public static bool SetExtraLatencyMode(ExtraLatencyMode mode)
Functions: Set current ExtraLatencyMode (Only call this once)
Parameter:
public enum ExtraLatencyMode
{
ExtraLatencyModeOff = 0, // Disable ExtraLatencyMode mode. This option will display the latest rendered frame for display.
ExtraLatencyModeOn = 1, // Enable ExtraLatencyMode mode. This option will display one frame prior to the latest rendered frame.
ExtraLatencyModeDynamic = 2 // Use system default setup.
}
Return value: true: Success,false: Failure
Method of calling: PXR_System.SetExtraLatencyMode(ExtraLatencyMode.ExtraLatencyModeOff);
7.8 System related¶
Through the relevant interfaces of System edition, developers can obtain and set some system configurations on Pico system edition devices
Supporting equipment:
G2 4K / G2 4K E (PUI version 3.11.3 and above);
Neo2 / Neo 2 Eye (PUI version 3.13.1 and above)
Neo 3 Pro / Neo 3 Pro Eye
Please initialize System Service before using the interfaces in this chapter. Detailed description as follow:
// Initializing and bind Service, the objectname refers to name of the object which is used to receive callback.
private void Awake()
{
PXR_System.InitSystemService(objectName);
PXR_System.BindSystemService();
}
// Unbind the Service
private void OnDestory()
{
PXR_Plugin.System.UnBindSystemService();
}
// Add 4 callback methods to allow corresponding callback can be received.
private void BoolCallback(string value)
{
if (PXR_Plugin.System.BoolCallback != null) PXR_Plugin.System.BoolCallback(bool.Parse(value));
PXR_Plugin.System.BoolCallback = null;
}
private void IntCallback(string value)
{
if (PXR_Plugin.System.IntCallback != null) PXR_Plugin.System.IntCallback(int.Parse(value));
PXR_Plugin.System.IntCallback = null;
}
private void LongCallback(string value)
{
if (PXR_Plugin.System.LongCallback != null) PXR_Plugin.System.LongCallback(int.Parse(value));
PXR_Plugin.System.LongCallback = null;
}
private void StringCallback(string value)
{
if (PXR_Plugin.System.StringCallback != null) PXR_Plugin.System.StringCallback(value);
PXR_Plugin.System.StringCallback = null;
}
Make sure Service is bound successfully before calling the interface. For callback of Service binding success, please add the following code to the initialization script. After Service binding success, this method will be called.
public void toBServiceBind(string s){Debug.Log("Bind success.");}
StateGetDeviceInfo¶
Function name: public static string UPxr_StateGetDeviceInfo(SystemInfoEnum type)
Functions: Get device info
Parameter: type - get the device info type
SystemInfoEnum.ELECTRIC_QUANTITY:Battery Power
SystemInfoEnum.PUI_VERSION:PUI Version
SystemInfoEnum.EQUIPMENT_MODEL:Device Model
SystemInfoEnum.EQUIPMENT_SN:Device SN code
SystemInfoEnum.CUSTOMER_SN:Customer SN code
SystemInfoEnum.INTERNAL_STORAGE_SPACE_OF_THE_DEVICE:Device Storage
SystemInfoEnum.DEVICE_BLUETOOTH_STATUS:Bluetooth Status
SystemInfoEnum.BLUETOOTH_NAME_CONNECTED:Bluetooth Name
SystemInfoEnum.BLUETOOTH_MAC_ADDRESS:Bluetooth MAC Address
SystemInfoEnum.DEVICE_WIFI_STATUS:Wi-Fi connection status
SystemInfoEnum.WIFI_NAME_CONNECTED:Connected Wi-Fi ID
SystemInfoEnum.WLAN_MAC_ADDRESS:WLAN MAC Adress
SystemInfoEnum.DEVICE_IP:Device IP Address
Return value: string - device info
Method of calling: PXR_Plugin.System.StateGetDeviceInfo(SystemInfoEnum.PUI_VERSION);
ControlSetAutoConnectWIFI¶
Function name: public static void ControlSetAutoConnectWIFI(string ssid, string pwd,Action<bool> callback)
Functions: Connect to the specified WiFi
Parameter: ssid: WiFi name, pwd: WiFi password, callback: successful/failed connection
Return value: None
Method of calling: PXR_Plugin.System.ControlSetAutoConnectWIFI(ssid, pwd,callback);
ControlClearAutoConnectWIFI¶
Function name: public static void ControlClearAutoConnectWIFI(Action<bool> callback)
Functions: Clear the specified WiFi
Parameter: callback: successful/failed clearance
Return value: None
Method of calling: PXR_Plugin.System.ControlClearAutoConnectWIFI(callback);
PropertySetHomeKey¶
Function name: public static void PropertySetHomeKey(HomeEventEnum eventEnum, HomeFunctionEnum function, Action<bool> callback)
Functions: Set Home key. It will redefine Home key and affect the function of Home key defined by the system. Please use this function with discretion.
Parameter: eventEnum: single click, double click and long press event
HomeEventEnum.SINGLE_CLICK:Single Click
HomeEventEnum.DOUBLE_CLICK:Double Click
HomeEventEnum.LONG_PRESS:Long Press
function: start setting apps, return, calibration, etc.
HomeFunctionEnum.VALUE_HOME_GO_TO_SETTING:Open Settings
HomeFunctionEnum.VALUE_HOME_BACK:Back Home App
HomeFunctionEnum.VALUE_HOME_RECENTER:Recenter
HomeFunctionEnum.VALUE_HOME_OPEN_APP:Open Defined App
HomeFunctionEnum.VALUE_HOME_DISABLE:Disable Home Button
HomeFunctionEnum.VALUE_HOME_GO_TO_HOME:Back to PUI Launcher
HomeFunctionEnum.VALUE_HOME_SEND_BROADCAST:Send Home Button Broadcast
HomeFunctionEnum.VALUE_HOME_CLEAN_MEMORY:Clear Background Process
callback: successful/failed
Return value: None
Method of calling: PXR_Plugin.System.PropertySetHomeKey(eventEnum,function,callback);
PropertySetHomeKeyAll¶
Function name: public static void PropertySetHomeKeyAll(HomeEventEnum eventEnum, HomeFunctionEnum function, int timesetup, string pkg, string className, Action<bool> callback)
Functions: Extended settings for Home key
Parameter: eventEnum: single click, double click and long press event
HomeEventEnum.SINGLE_CLICK:Single Click
HomeEventEnum.DOUBLE_CLICK:Double Click
HomeEventEnum.LONG_PRESS:Long Press
function start setting apps, return, calibration, etc.
HomeFunctionEnum.VALUE_HOME_GO_TO_SETTING:Open Settings
HomeFunctionEnum.VALUE_HOME_BACK:Back Home App
HomeFunctionEnum.VALUE_HOME_RECENTER:Recenter
HomeFunctionEnum.VALUE_HOME_OPEN_APP:Open Defined App
HomeFunctionEnum.VALUE_HOME_DISABLE:Disable Home Button
HomeFunctionEnum.VALUE_HOME_GO_TO_HOME:Back to PUI Launcher
HomeFunctionEnum.VALUE_HOME_SEND_BROADCAST:Send Home Button Broadcast
HomeFunctionEnum.VALUE_HOME_CLEAN_MEMORY:Clear Background Process
timesetup: The interval of key pressing is set only if there are events when double clicking or long pressing event. When short pressed, it will return 0.
pkg: When Function is HOME_FUNCTION_OPEN_APP, input specified package name; other situations input null.
className: When Function is HOME_FUNCTION_OPEN_APP, input specified class name; other situations input null.
callback: succeed/ failed
Return value: None
Method of calling: PXR_Plugin.System.UPxr_PropertySetHomeKeyAll(eventEnum,function, timesetup, pkg, className, callback);
PropertyDisablePowerKey¶
Function name: public static void PropertyDisablePowerKey(bool isSingleTap, bool enable,Action<int> callback)
Functions: Set power key
Parameter: isSingleTap: single click event [true], long press event [false], enable: key enabling status; callback: successful/failed setting
Return value: None
Method of calling: PXR_Plugin.System.PropertyDisablePowerKey(isSingleTap, enable,callback);
PropertySetScreenOffDelay¶
Function name: public static void PropertySetScreenOffDelay(ScreenOffDelayTimeEnum timeEnum,Action<int> callback)
Functions: Set screen off delay, please note that screen off timeout should not be larger than system sleep timeout
Parameter: timeEnum: screen off delay
ScreenOffDelayTimeEnum. THREE:3 Seconds
ScreenOffDelayTimeEnum. TEN:10 Seconds
ScreenOffDelayTimeEnum. THIRTY:30 Seconds
ScreenOffDelayTimeEnum. SIXTY:60 Seconds
ScreenOffDelayTimeEnum. THREE_HUNDRED:5 Minutes
ScreenOffDelayTimeEnum. SIX_HUNDRED:10 Minutes
ScreenOffDelayTimeEnum. NEVER:Never Turn Screen Off
callback: successful/failed setting
Return value: None
Method of calling: PXR_Plugin.System.PropertySetScreenOffDelay(timeEnum,callback);
PropertySetSleepDelay¶
Function name: public static void PropertySetSleepDelay(SleepDelayTimeEnum timeEnum)
Functions: Set sleep delay
Parameter: timeEnum: system sleep delay
SleepDelayTimeEnum. FIFTEEN:15 Seconds
SleepDelayTimeEnum. THIRTY:30 Seconds
SleepDelayTimeEnum. SIXTY:60 Seconds
SleepDelayTimeEnum. THREE_HUNDRED:5 Minutes
SleepDelayTimeEnum. SIX_HUNDRED:10 Minutes
SleepDelayTimeEnum. ONE_THOUSAND_AND_EIGHT_HUNDRED:30 Minutes
SleepDelayTimeEnum. NEVER:Never Sleep
Return value: None
Method of calling: PXR_Plugin.System.PropertySetSleepDelay(timeEnum);
SwitchSystemFunction¶
Function name: public static void SwitchSystemFunction(SystemFunctionSwitchEnum systemFunction, SwitchEnum switchEnum)
Functions: Set switch system funtion
Parameter: systemFunction: type of function, switchEnum: switch value
SystemFunctionSwitchEnum.SFS_USB:USB Debugging
SystemFunctionSwitchEnum.SFS_AUTOSLEEP:Auto Sleep
SystemFunctionSwitchEnum.SFS_SCREENON_CHARGING:Screen-On Charging
SystemFunctionSwitchEnum.SFS_OTG_CHARGING:OTG Charging
SystemFunctionSwitchEnum.SFS_RETURN_MENU_IN_2DMODE:Return Button In 2D Mode
SystemFunctionSwitchEnum.SFS_COMBINATION_KEY:Key Combination
SystemFunctionSwitchEnum.SFS_CALIBRATION_WITH_POWER_ON:Recenter/Re-Calibration On Device Boot
SystemFunctionSwitchEnum.SFS_SYSTEM_UPDATE:System Update
SystemFunctionSwitchEnum.SFS_CAST_SERVICE:Casting Service (This Property is not valid when using Pico Enterprise Solution)
SystemFunctionSwitchEnum.SFS_EYE_PROTECTION:Eye-Protection Mode
SystemFunctionSwitchEnum.SFS_SECURITY_ZONE_PERMANENTLY:Disable 6Dof Safety Boundary Permanently
SystemFunctionSwitchEnum.SFS_GLOBAL_CALIBRATION:Global Recenter/Re-Calibrate (Only available for G2 series)
SystemFunctionSwitchEnum.SFS_Auto_Calibration:Auto Recenter/Re-Calibrate
SystemFunctionSwitchEnum.SFS_USB_BOOT:USB Plug-in Boot
switchEnum: switch value
SwitchEnum.S_ON:On
SwitchEnum.S_OFF:Off
Method of calling: PXR_Plugin.System.SwitchSystemFunction(systemFunction,switchEnum);
SwitchSetUsbConfigurationOption¶
Function name: public static void SwitchSetUsbConfigurationOption(USBConfigModeEnum uSBConfigModeEnum)
Functions: Set USB configuration (MTP, charging )
Parameter: uSBConfigModeEnum: MTP. charging
USBConfigModeEnum.MTP:MTP Mode
USBConfigModeEnum.CHARGE:Charging Mode
Return value: None
Method of calling: PXR_Plugin.System.SwitchSetUsbConfigurationOption(uSBConfigModeEnum);
AcquireWakeLock¶
Function name: public static void AcquireWakeLock()
Functions: Acquire wakelock
Parameters: None
Return value: None
Method of calling: PXR_Plugin. System.AcquireWakeLock ();
ReleaseWakeLock¶
Function name: public static void ReleaseWakeLock()
Functions: Release wakelock
Parameters: None
Return value: None
Method of calling: PXR_Plugin. System.ReleaseWakeLock ();
EnableEnterKey¶
Function name: public static void EnableEnterKey()
Functions: Enable Confirm key
Parameters: None
Return value: None
Method of calling: PXR_Plugin. System.EnableEnterKey();
DisableEnterKey¶
Function name: public static void DisableEnterKey()
Functions: Disable Confirm key
Parameters: None
Return value: None
Method of calling: PXR_Plugin. System.DisableEnterKey();
EnableVolumeKey¶
Function name: public static void EnableVolumeKey()
Functions: Enable Volume key
Parameters: None
Return value: None
Method of calling: PXR_Plugin.System.EnableVolumeKey();
DisableVolumeKey¶
Function name: public static void DisableVolumeKey()
Functions: Disable Volume key
Parameters: None
Return value: None
Method of calling: PXR_Plugin.System.DisableVolumeKey();
EnableBackKey¶
Function name: public static void EnableBackKey()
Functions: Enable Back key
Parameters: None
Return value: None
Method of calling: PXR_Plugin.System.EnableBackKey();
DisableBackKey¶
Function name: public static void UPxr_DisableBackKey()
Functions: Disable Back key
Parameters: None
Return value: None
Method of calling: PXR_Plugin.System.DisableBackKey();
WriteConfigFileToDataLocal¶
Function name: public static void UPxr_WriteConfigFileToDataLocal(string path, string content, Action<bool> callback)
Functions: Write configuration file to Data/local/tmp
Parameters: path: configure file path (“/data/local/tmp/config.txt”), content: configure file conten(“” ), callback: whether the configuration file has been successfully written
Return value: None
Method of calling: PXR_Plugin.System. WriteConfigFileToDataLocal(string path, string content, Action<bool> callback);
ResetAllKeyToDefault¶
Function name: public static void ResetAllKeyToDefault (Action<bool> callback)
Functions: Reset all keys to default
Parameters: callback: whether all keys have been successfully reset to default
Return value: None
Method of calling: PXR_Plugin.System. ResetAllKeyToDefault (Action<bool> callback);
OpenMiracast (New)¶
Function name: public static void OpenMiracast()
Functions: Turn on the screencast function.
Parameter: None
Return value: None
Method of calling: PXR_System. OpenMiracast();
IsMiracastOn (New)¶
Function name: public static void IsMiracastOn()
Functions: Get the status of the screencast.
Parameter: None
Return value: true: on; false: off
Method of calling: PXR_System. IsMiracastOn();
CloseMiracast (New)¶
Function name: public static void CloseMiracast()
Function: Turn off the screencast function.
Parameter: None
Return value: None
Method of callilng: PXR_System. CloseMiracast();
StartScan (New)¶
Function name: public static void StartScan()
Functions: Start scanning for devices that can be screened.
Parameter: None
Return value: None
Method of calling: PXR_System. StartScan();
StopScan (New)¶
Function name: public static void StopScan()
Functions: Stop scanning for devices that can be screened.
Parameter: None
Return value: None
Method of calling: PXR_System. StopScan();
SetWDJsonCallback (New)¶
Function name: public static void SetWDJsonCallback()
Function: Set callback of scanning result, return a string in json format, including devices connected and scanned before.
Parameter: None
Return value: None
Method of calling: PXR_System. SetWDJsonCallback();
ConnectWifiDisplay (New)¶
Function name: public static void ConnectWifiDisplay(string modelJson)
Functions: Project to the specified device.
Parameter: modelJson
{
"deviceAddress": "e2:37:bf:76:33:c6",
"deviceName": "\u5BA2\u5385\u7684\u5C0F\u7C73\u7535\u89C6",
"isAvailable": "true",
"canConnect": "true",
"isRemembered": "false",
"statusCode": "-1",
"status": "",
"description": ""
}
Return value: None
Method of calling: PXR_System. ConnectWifiDisplay(modelJson);
DisConnectWifiDisplay (New)¶
Function name: public static void DisConnectWifiDisplay()
Functions: Disconnected for screencast.
Parameter: None
Return value: None
Method of calling: PXR_System. DisConnectWifiDisplay();
ForgetWifiDisplay (New)¶
Function name: public static void ForgetWifiDisplay(string address)
Functions: Forget the devices that have been connected.
Parameter: address: the adress of the device.
Return value: None
Method of calling: PXR_System. ForgetWifiDisplay(address);
RenameWifiDisplay (New)¶
Function name: public static void RenameWifiDisplay (string address, string newName)
Functions: Modify the name of the connected device (only the name for local storage)
Parameter:address: the adress of the device; newName: target name of the device.
Return value: None
Method of calling: PXR_System. RenameWifiDisplay (address, newName);
UpdateWifiDisplays (New)¶
Function name: public static void UpdateWifiDisplays(Action<string>callback)
Functions: Manually update the list.
Parameter: callback: the result of the SetWDJsonCallback.
Return value: None
Method of calling: PXR_System. UpdateWifiDisplays(callback);
GetConnectedWD (New)¶
Function name: public static string GetConnectedWD()
Functions: Get the information of the currently connected device.
Parameter: None
Return value: Return the currently connected device information.
Method of calling: PXR_System. GetConnectedWD();
7.8.2 Protected interfaces¶
Note: To call the following interfaces, it is required to add this label in the manifest. If this label is added, the app will not be available in the Pico Store.
<meta-data android:name="pico_advance_interface" android:value="0"/>
ControlSetDeviceAction¶
Function name: public static void ControlSetDeviceAction(DeviceControlEnum deviceControl,Action<int> callback)
Functions: Make the device to shut down or reboot
Parameter: deviceContro: Make the device to shut down or reboot
DeviceControlEnum.DEVICE_CONTROL_REBOOT:Reboot
DeviceControlEnum.DEVICE_CONTROL_SHUTDOWN:Power Off
callback: callback interface, successful/failed shut down/ reboot
Return value: None
Method of calling: PXR_Plugin.System.ControlSetDeviceAction(DeviceControlEnum.DEVICE_CONTROL_SHUTDOWN,callback);
ControlAPPManger¶
Function name: public static void ControlAPPManger(PackageControlEnum packageControl, string path, Action<int> callback)
Functions: Silently install or uninstall app
Parameter: packageControl: Silent installation/uninstallation
PackageControlEnum.PACKAGE_SILENCE_INSTALL:Install
PackageControlEnum.PACKAGE_SILENCE_UNINSTALL:Uninstall
path: The path to the installation package for the silent installation or the name of the app package when silently uninstalled; callback: callback interface, successful/ failed installation/ uninstallation
Return value: None
Method of calling: PXR_Plugin.System.ControlAPPManger(PackageControlEnum.PACKAGE_SILENCE_UNINSTALL, “com.xxx.xxx”,callback);
ScreenOn¶
Function name: public static void ScreenOn()
Functions: Turn screen on
Parameter: None
Return value: None
Method of calling: PXR_Plugin.System.ScreenOn();
ScreenOff¶
Function name: public static void ScreenOff()
Functions: Turn screen off
Parameter: None
Return value: None
Method of calling: PXR_Plugin.System. ScreenOff()
KillAppsByPidOrPackageName (New)¶
Function name: public static void KillAppsByPidOrPackageName(int[] pids, string[] packageNames)
Functions: Kill the application by passing in the application pid or package name.
Parameter: pid: an array of application pid, packageNames: an array of package names.
Return value: None
Method of calling: PXR_System. KillAppsByPidOrPackageName(pids, packageNames);
7.9 Achievement system related¶
7.9.1 Preparations¶
To access the Achievement system, developers need to create the application on Pico Developer Platform and obtain APP ID. Steps:
- Log on to Pico Developer Platform (https://developer.pico-interactive.com/) and sign up for a Pico membership
- Apply to be a developer
Developers are divided into individual developers and enterprise developers, please apply according to the actual situation. After submission, we will give feedback within 3 working days. Please check the status in time.
- View Merchant ID
- Create Achievement
(1). Click “View” for more operations. Creating first if you don’t have any App. After successful creation, the platform will assign an unique APP ID automatically.
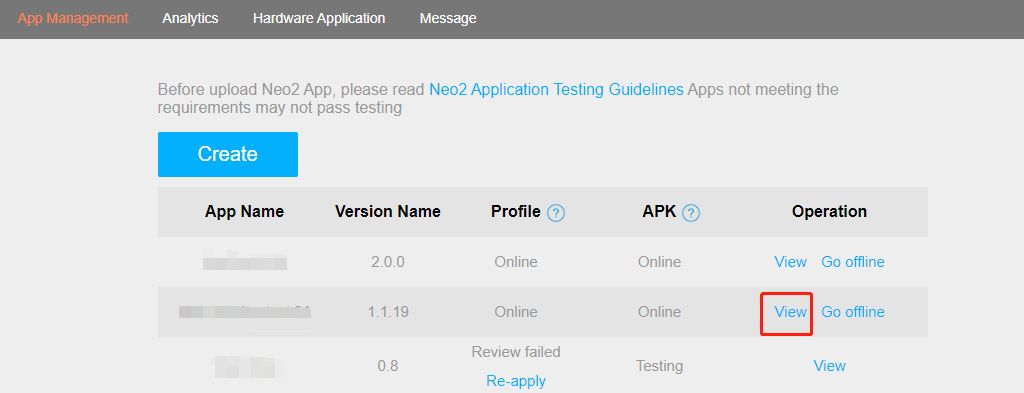
Fig 7.5 Pico Developer Platform
(2). Click the button of “Platform Service - Achievements” at the bottom of the View page.
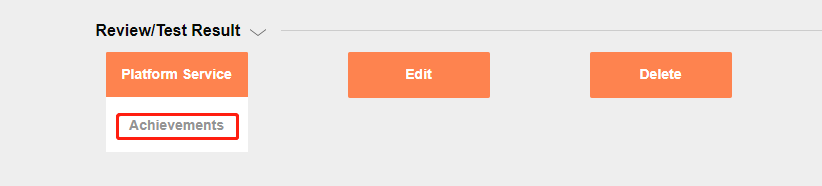
Fig 7.6 Pico Developer Platform
(3). Checking the list of achievements you have created. Click “Create” if you don’t have any achievement.
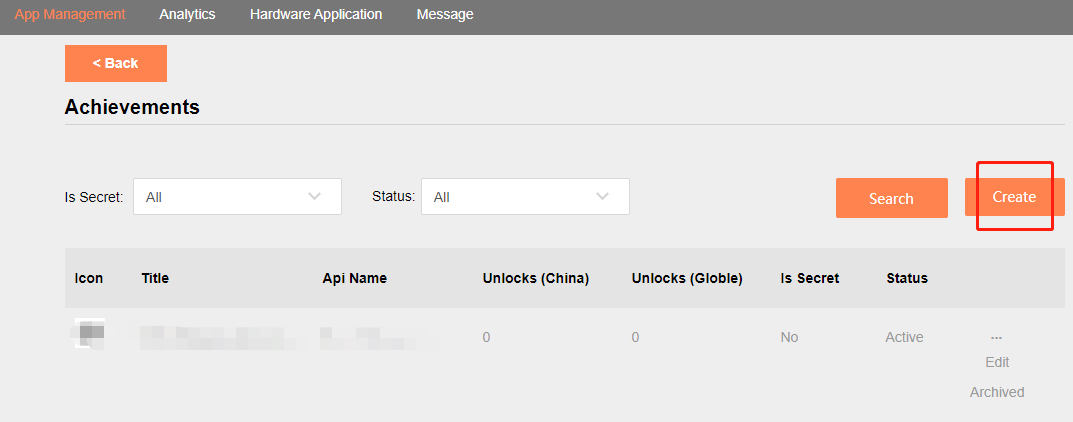
Fig 7.7 Pico Developer Platform
(4). Fill in the fields of your achievement. Write the same API Name of that in your coding.
- Achievement types:
(1). Simple: Unlock by completing a single event or target without achievement progress
(2). Counter: Unlock when the specified number of targets is reached (e.g. reach 5 targets to unlock, Target = 5)
(3). Bitfield: Unlock when the specified number of targets in the specified range is reached (e.g. reach 3 out of 7 targets to unlock, Target = 3, Bitfield Length = 7)
- Configure App ID
(1).Configure a valid APP ID in the project Platform Settings and Manifest.xml:
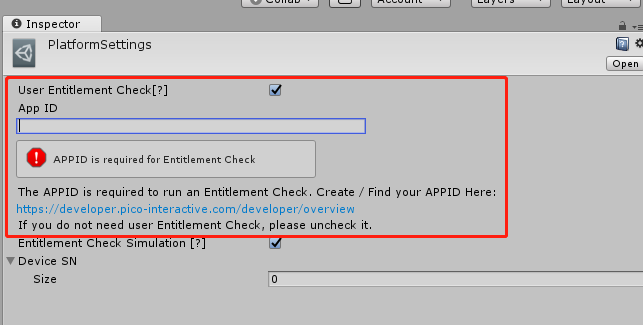
Fig 7.8 Platform Settings panel
(2). See Chapter 9.1.1 and Chapter 9.1.2 for detailed AndroidManifest configuration.
7.9.2 Calling interfaces¶
Init¶
Function name: public static PXR_Request<PXR_AchievementUpdate> Init()
Functions: Achievement system initialization
Parameters: None
Return value: Result of calling
Method of calling: PXR_Achievement.Init()
GetAllDefinitions¶
Function name: public static PXR_Request<PXR_AchievementDefinitionList> GetAllDefinitions()
Functions: Get all definitions of achievements
Parameters: None
Return value: Result of calling
Method of calling: PXR_Achievement. GetAllDefinitions ()
GetAllProgress¶
Function name: public static PXR_Request<PXR_AchievementProgressList> GetAllProgress()
Functions: Get progress of all modified achievements
Parameters: None
Return value: Result of calling
Method of calling: PXR_Achievement. GetAllProgress ()
GetDefinitionsByName¶
Function name: public static PXR_Request<PXR_AchievementDefinitionList> GetDefinitionsByName(string[] names)
Functions: Get definitions by name
Parameters: names: get the achievement apiname for obtaining definitions
Return value: Result of calling
Method of calling: PXR_Achievement. GetDefinitionsByName (names)
GetProgressByName¶
Function name: public static PXR_Request<PXR_AchievementProgressList> GetProgressByName(string[] names)
Functions: Get achievement progress by name
Parameters: names: the achievement apiname for obtaining definitions
Return value: Result of calling
Method of calling: PXR_Achievement. GetProgressByName (names)
AddCount¶
Function name: public static PXR_Request<PXR_AchievementUpdate> AddCount(string name, long count)
Functions: Add count achievements
Parameters: name: achievement apiname,count: additions
Return value: Result of calling
Method of calling: PXR_Achievement. AddCount (name,count)
AddFields¶
Function name: public static PXR_Request<PXR_AchievementUpdate> AddFields(string name, string fields)
Functions: Add bitfield achievements
Parameters: name: achievement apiname, fields: additions
Return value: Result of calling
Method of calling: PXR_Achievement. AddFields (name,bitfield)
Unlock¶
Function name: public static PXR_Request<PXR_AchievementUpdate> Unlock(string name)
Functions: Unlock achievement according to apiname
Parameters: name: achievement apiname
Return value: Result of calling
Method of calling: PXR_Achievement. Unlock (name)
GetNextAchievementDefinitionListPage¶
Function name: public static PXR_Request<PXR_AchievementDefinitionList> GetNextAchievementDefinitionListPage(PXR_AchievementDefinitionList list)
Functions: When getting all the achievement definitions, if there are multiple pages, get the next page
Parameters: None
Return value: Result of calling
Method of calling: PXR_Achievement. GetNextAchievementDefinitionListPage(achievementDefinitionList)
GetNextAchievementProgressListPage¶
Function name: public static PXR_Request<PXR_AchievementProgressList> GetNextAchievementProgressListPage(PXR_AchievementProgressList list)
Functions: When getting all modified achievement progress information, if there are multiple pages, get the next page
Parameters: None
Return value: Result of calling
Method of calling: PXR_Achievement. GetNextAchievementProgressListPage(achievementProgressList)
7.9.3 Notes¶
(1). Please log in to the device with Pico account first, and then use the achievement interface and functions
(2). To avoid errors in achievement data caused by account switching and logout, please call initialization again in App Resume() to ensure that the App gets the correct user login information.
(3). At the development and debugging phase, the following method can be adopted to debug and test
- Logcat
- View the data from the Developer Platform - Apps - Achievements page