7 API interface function list¶
7.1 General function library¶
The SDK supports VR general functions indicated by the red dots in the following engine:
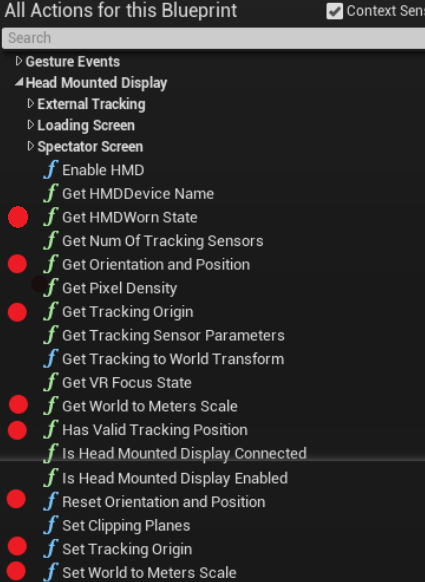
Figure 7.1 Supported general functions
For their detailed usage, please refer to the official UE4 document at
https://docs.unrealengine.com/4.27/en-US/
Among them:
1. Reset Orientation and Position nodes only support the function of resetting the positive direction, and the Yaw parameter values in the function node does not work.
2. Using Get HMDWorn State requires enabling option “Enable Psensor” in Project Setting - PicoXR Settings
7.2 Dedicated function library¶
For system functions such as volume and brightness, the SDK also provides the corresponding APIs as blueprint nodes. Right-click on the event graph to find APIs in Pico Mobile category:
The detailed instructions for APIs are as follows:
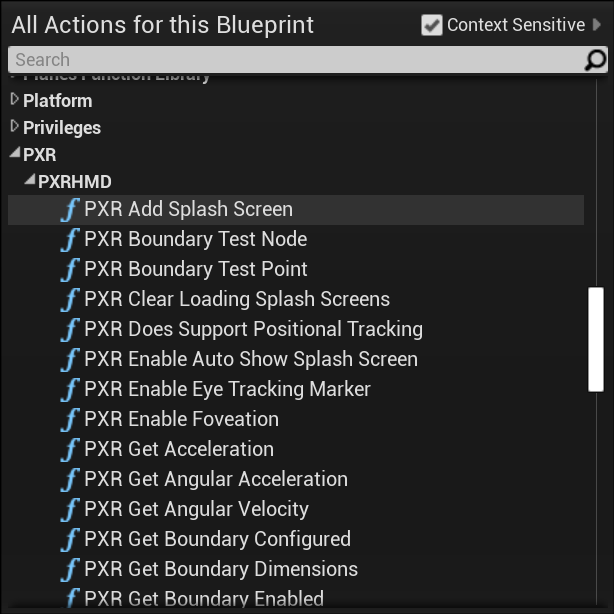
Figure 7.2 HMD dedicated function library
PXR Get Current Orientation¶
Get the current orientation of the headset, return value is Quat
Blueprint:
Input: None
Output: None
Return:
- Quat:
- Current orientation of the headset
PXR Get Device Model¶
Get the model of the device,e.g. “Pico Neo 3”
Blueprint:
Input: None
Output: None
Return:
- FString:
- Current device model
PXR Get Field Of View¶
Get headset FOV in horizontal and vertical direction.
Blueprint:
Input: None
Output:
- Float:
- HFOVIn Degrees: horizontal FOV, unit is degree
- VFOVIn Degrees: vertical FOV, unit is degree
Return:
- Bool:
- true: Success
- false: Failure
PXR Does Support Positional Tracking¶
Check if headset supports positional tracking. Returns true when 6Dof mode is enabled, and false if 3Dof mode
Blueprint:
Input: None
Output: None
Return:
- Bool:
- true: Support positional tracking(6DOF)
- false: Not support positional tracking(3DOF)
PXR Get Current Display Frequency¶
Get current screen display refresh rate. Currently 72Hz, 90Hz, 120Hz are supported. Default is 72Hz. 90Hz and 120Hz needs to be setup in Settings - Lab.
Blueprint:
Input: None
Output: None
Return:
- Float:
- 0: Failed to acquire refresh rate
- 72: 72hz Mode
- 90: 90hz Mode
- 120: 120hz Mode
PXR Get HMDWorn State¶
Get current headset worn state, including Worn, NotWorn, Unknown states. Needs to be set in Plugins - PicoXR Settings. “Enable PSensor” option requires to be enabled. Retunrs Unknown if it’s not checked.
Blueprint:
Input: None
Output: None
Return:
- Enum:
- EHMDWornState:
- Unknown: Enable PSensor disabled,or PSensor detection failed
- Worn: Enable PSensor enabled,and PSensor is occuluded
- NotWorn: Enable PSensor enabled,and PSensor is not occuluded
- EHMDWornState:
PXR Reset HMDSensor¶
Reset headset orientation and position. Similar to Reset Orientation and Position.
Blueprint:
Input: None
Output: None
Return:
- Bool:
- true: Success
- false: Failure
PXR Set CPUAndGPULevels¶
Set CPU and GPU clock frequency. Available levels are 1/2/3/4/5 where 1 is slowest and battery-saving. Higher levels increase performance but generate more heats and battery consumption.
Blueprint:
Input:
- Int:
- CPU Level: CPU clock frequency level,Int: 1 to 5.
- GPU Level: GPU clock frequency level,Int: 1 to 5.
Output: None
Return: None
PXR Get IPD¶
Get IPD value of current device.
Blueprint:
Input: None
Output: None
Return:
- Float:
- Current IPD
PXR IPDChangedDelegates¶
Bind IPD changing delegate event. Can be used to update new IPD
Blueprint:
Input:
Delegate:
- On Pico XRIPDChanged: bind IPD changing callback event as shown below:
- Callback params:
- Float:
- Ipd: Current IPD value
- Float:
Output: None
Return: None
PXR Get Event Manager¶
Use blueprint type to acquire current event manager and event manager can be used to bind callback event to delegate. Current support controller connection delegate, IPD change delegate, Long Home key pressing, Refresh Rate Changed Delegate, Resume Delegate.
Blueprint:
Input: None
Output: None
Return:
Reference to singleton of event manager
Each delegate binding method as follow:
Device Connect Changed Delegate¶
Use delegate to bind event for controller connection state change and acquire parameter change, e.g. Handness being 0 and State being 1 indicates Left controller is connected
Blueprint:
Callback event parameter:
- Int:
- Handness: Controller ID:
- 0: Left controller
- 1: Right controller
- State connection state:
- 0: Connecion lost
- 1: Connection established
- Handness: Controller ID:
Ipd Changed Delegate¶
Use delegate to bind IPD to change events and acquire IPD value after changing.
Blueprint:
Callback event params:
- Float:
- New Ipd: IPD after being changed
Refresh Rate Changed Delegate¶
Use delegate to bind refresh rate changing event and acquire refresh rate after the update.
Blueprint:
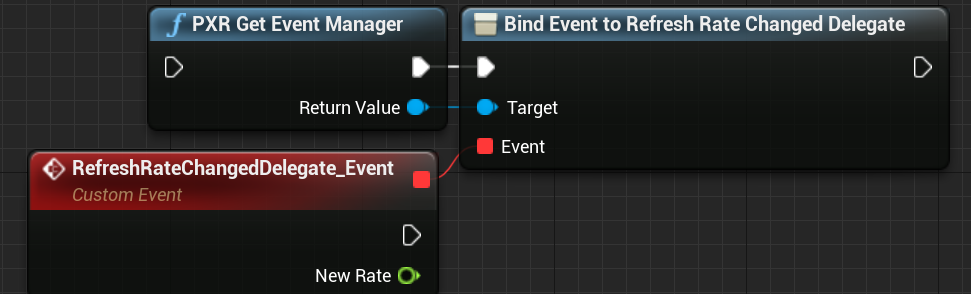
Callback event params:
- Float:
- New Rate: Refresh rate after update
Resume Delegate¶
Use delegate to bind resume event. For example, when pressing home event and returns to main menu. Then pressing home event to return to game to trigger this event.
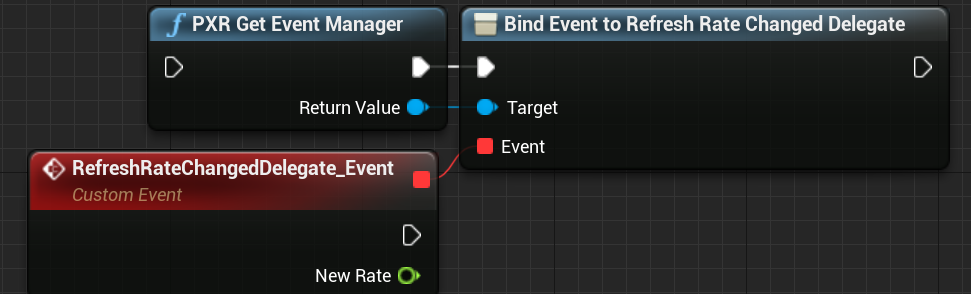
PXR Set See Through Background¶
Set See Through images as camera background. Note: Not applicable for UE 4.24 and 4.25. Applicable for 4.26, 4.27.
Blueprint:
Input:
- Bool:
- true: Enable camera images as background
- false: Disable using camera images as background
Output: None
Return:
- Int:
- 0: Success
- -1: Failure
PXR Get Dialog State¶
Get dialog state of Safety Boundary
Blueprint:
Input: None
Output: None
Return:
- Enum:
- State(EPicoXRBoundaryState):
- GobackDialog: Visible when leaving safety boundary, hidden when back to safety boundary
- ToofarDialog: Away from safety boundary for over 3m
- LostDialog: 6Dof lost with no reason
- LostNoReason: 6Dof lost with no reason
- LostCamera: Camera caliberation params incorrect
- LostHighLight: Too bright
- LostLowLight: Too dark
- LostLowFeatureCount: Not enough environment feature points
- LostReLocation: Recovering positional tracking
- LostInitialization: Initializing boundary
- LostNoCamera: Camera data incorrect
- LostNoIMU: IMU data incorrect
- LostIMUJitter: IMU data jitter
- LostUnknown: Unknown lost
- NothingDialog: Positional tracking disabled with no Dialog
- State(EPicoXRBoundaryState):
7.3 Input relevent libary¶
The following APIs are available in PXRInput category:
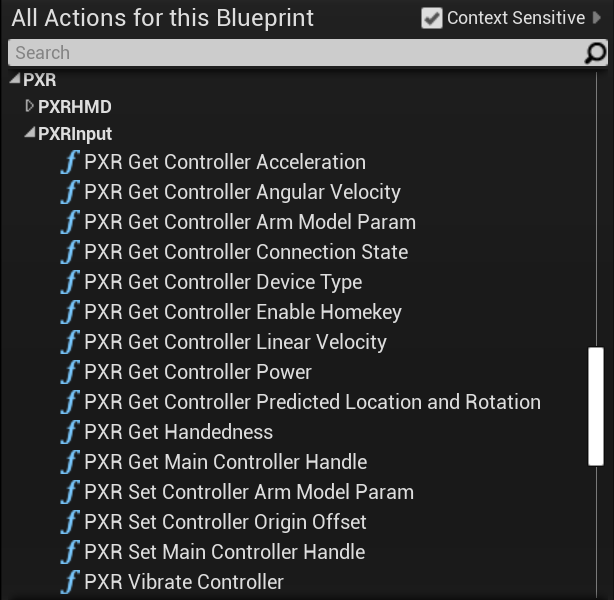
Figure 7.3 Input relevent libary
PXR Get Controller Device¶
Get controller type of connected controller
Blueprint:
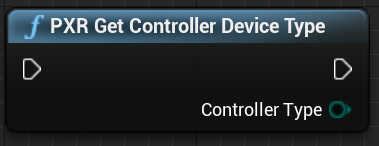
Input: None
Output:
- Enum:
- Controller Type(EPicoXRControllerDeviceType):
- UnKnown: Unkown controller
- Neo3: Pico Neo3 controller
- Controller Type(EPicoXRControllerDeviceType):
Return: None
PXR Get Controller Power¶
Get controller power
Blueprint:
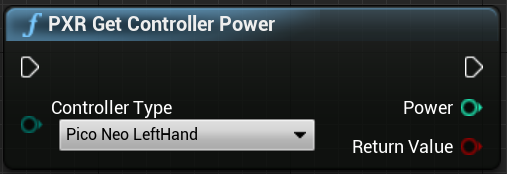
Input:
- Enum:
- Controller Type(EPicoXRControllerType):
- Pico Neo LeftHand: Left controller
- Pico Neo RightHand: Right controller
- Controller Type(EPicoXRControllerType):
Output:
- Int:
- Power: Power of assigned controller (range: 1-5)
Return:
- Bool:
- true: Success
- false: Failure
PXR Get Controller Connection State¶
Get controller connection state of specified controller
Blueprint:
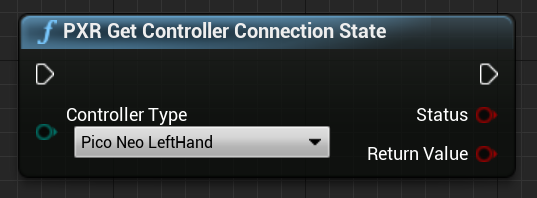
Input:
- Enum:
- Controller Type(EPicoXRControllerType):
- Pico Neo LeftHand: Left controller
- Pico Neo RightHand: Right controller
- Controller Type(EPicoXRControllerType):
Output:
- Bool:
- true: Connected
- false: Not connected
Return:
- Bool:
- true: Success
- false: Failure
PXR Vibrate Controller¶
Manipulate controller vibration
Blueprint:
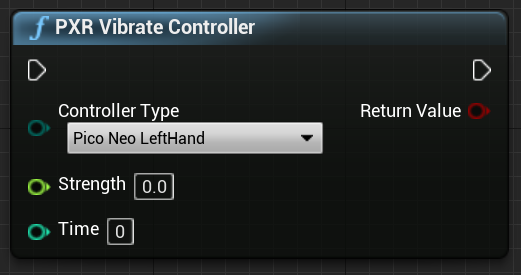
Input:
- Enum:
- Controller Type(EPicoXRControllerType):
- Pico Neo LeftHand: Left controller
- Pico Neo RightHand: Right controller
- Controller Type(EPicoXRControllerType):
- Float:
- Strength: Vibration strength (Range: 0-1)
- Int:
- Time: Vibration strength(unit is ms,Range: 0-65535)
Output: None
Return:
- Bool:
- true: Success
- false: Failure
PXR Get Controller Acceleration¶
Get controller acceleration of specified controller
Blueprint:
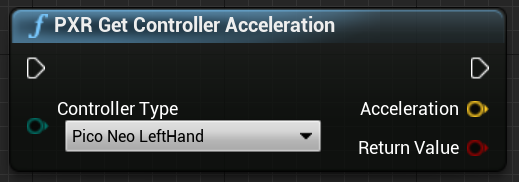
Input:
- Enum:
- Controller Type(EPicoXRControllerType ):
- Pico Neo LeftHand: Left controller
- Pico Neo RightHand: Right controller
- Controller Type(EPicoXRControllerType ):
Output:
- FVector:
- Acceleration: Controller acceleration(Unit: cm/s)
Return:
- Bool:
- true: Success
- false: Failure
PXR Get Controller Angular Velocity¶
Get controller angular velocity of specified controller
Blueprint:
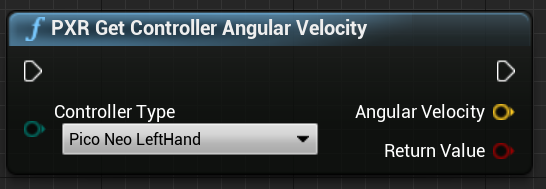
Input:
- Enum:
- Controller Type(EPicoXRControllerType):
- Pico Neo LeftHand: Left controller
- Pico Neo RightHand: Right controller
- Controller Type(EPicoXRControllerType):
Output:
- FVector:
- Angular Velocity: Controller angular velocity(rad/s)
Return:
- Bool:
- true: Success
- false: Failure
PXR Get Controller Linear Velocity¶
Get linear velocity of specified controller
Blueprint:
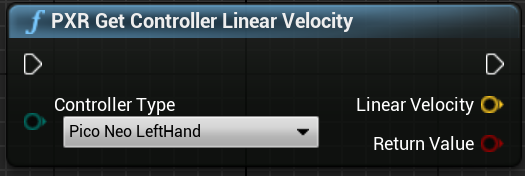
Input:
- Enum:
- Controller Type(EPicoXRControllerType):
- Pico Neo LeftHand: Left controller
- Pico Neo RightHand: Right controller
- Controller Type(EPicoXRControllerType):
Output:
- FVector:
- Linear Velocity: Controller linear velocity(cm/s)
Return:
- Bool:
- true: Success
- false: Failure
PXR Set Controller Origin Offset¶
Set controller origin offset of specified controller
Blueprint:
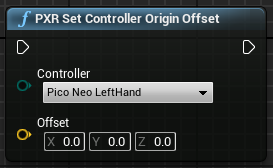
Input:
- Enum:
- Controller Type(EPicoXRControllerType):
- Pico Neo LeftHand: Left controlller
- Pico Neo RightHand: Right controller
- Controller Type(EPicoXRControllerType):
- Vector:
- Offset: Controller origin offset(Unit: Meter)
Output: None
Return: None
PXR Get Controller Predicted Location and Rotation¶
Get controller predicted location and rotation of specified hand
Blueprint:
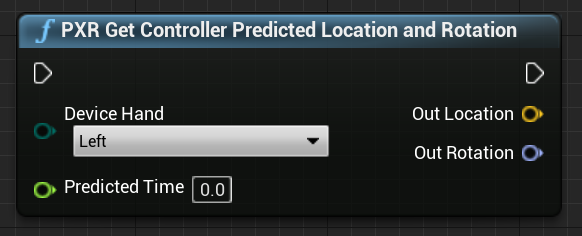
Input:
- Enum:
- Device Hand(EControllerHand):
- Left: Left controller
- Right: Right controller
- Device Hand(EControllerHand):
- Float:
- Predicted Time: Range: 0-100ms
Output:
- FVector:
- Location: Predicted controller location by given time
- FRotator:
- Rotation: Rotation: Predicted controller rotation by given time
Return: None
PXR Get Controller Enable Homekey¶
Get if controller home key is enabled
Blueprint:
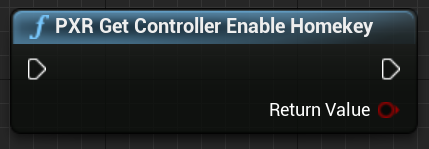
Input: None
Output: None
Return:
Bool:
- true: Enable listening tot home key events
- false: Disable listening tot home key events
7.4 Eye Tracking relevant library¶
The following APIs are available in PXRHMD category. Note: Before using Eye Tracking APIs, refer to chapter 8.2 for eye tracking relevant configurations.
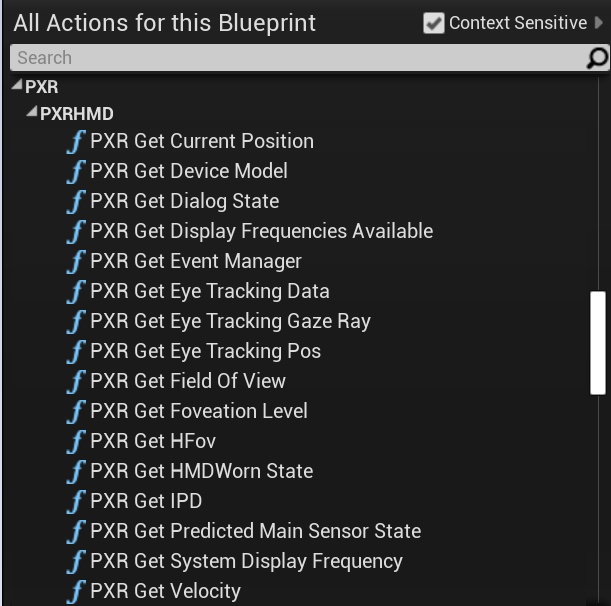
Figure 7.4 Eye Tracking relevant libary
PXR Get Eye Tracking Data¶
Get eye tracking data (Only supported on Neo 3 Pro Eye. Requires “Enable Eye Tracking” checked)
Blueprint:
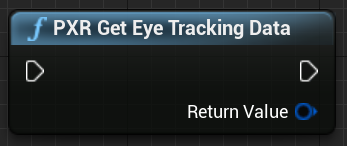
Input: None
Output: None
Return:
- Struct:
- FPicoXREyeTrackingData:
- Int32: LeftEyePoseStatus: Current left eye pose status
- 0: Data available
- -1: Data not available
- Int32: RightEyePoseStatus: Current right eye pose status
- 0: Data available
- -1: Data not available
- Int32: CombinedEyePoseStatus: Current combined eye pose status
- 0: Data available
- -1: Data not available
- FVector: CombinedEyeGazePoint: Current combined eye gaze point
- FVector: CombinedEyeGazeVector: Current combined eye gaze vector(Vector pointing from middle point of both eyes to gazing point)
- Float: LeftEyeOpenness: Get current left eye openness
- 0.0: Eye closed
- 1.0: Eye open
- Float: RightEyeOpenness: Get current right eye openness
- 0.0: Eye closed
- 1.0: Eye open
- FVector: LeftEyePositionGuide: Left eye position guide
- FVector: RightEyePositionGuide: Righr eye position guide
- FVector: FoveatedGazeDirection: Gazing ray direction (center of FFR implemented)
- Int32: LeftEyePoseStatus: Current left eye pose status
- FPicoXREyeTrackingData:
7.5 Foveation Rendering relevant libary¶
The following APIs are available in PXRHMD category. Note: Before using Foveation Rendering APIs, refer to chapter 8.3 for eye tracking relevant configurations.
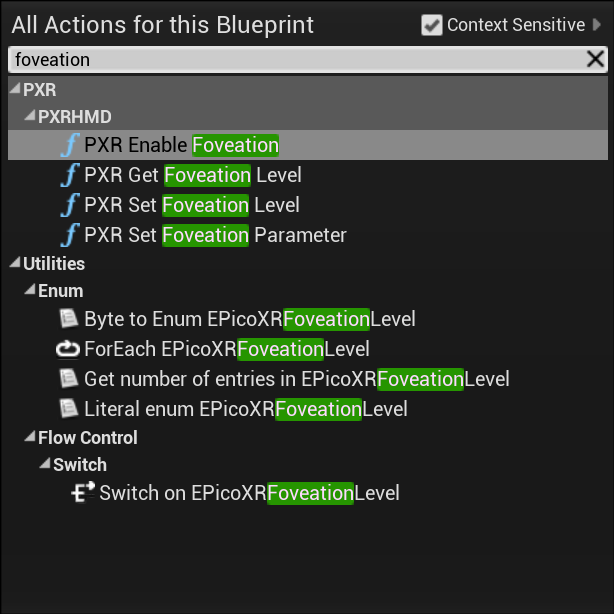
Figure 7.5 Foveation Rendering relevant libary
PXR Enable Foveation¶
Enable/Disable foveation rendering
Blueprint:
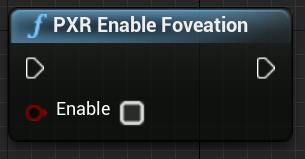
Input:
- Bool:
- Checked: true,Foveation rendering enabled
- Unchecked: false,Foveation rendering disbaled
Output: None
Return: None
PXR Get Foveation Level¶
Get current foveation rendering level
Blueprint:
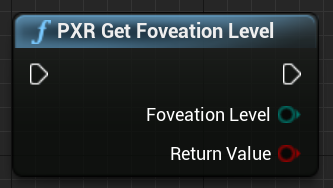
Input: None
Output:
- Enum:
- Foveation Level(EPicoXRFoveationLevel):
- Low
- Medium
- High
- TopHigh
- Foveation Level(EPicoXRFoveationLevel):
Return:
- Bool:
- true: Success
- false: Failure
PXR Set Foveation Level¶
After packaged, this interface can be used to set foveation level in runtime. (Note: “Enable FoveationRendering” has to be enabled before packaging)
Blueprint:
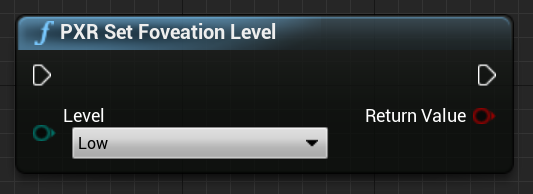
Input:
- Enum:
- Foveation Level(EPicoXRFoveationLevel):
- Low
- Medium
- High
- TopHigh
- Foveation Level(EPicoXRFoveationLevel):
Output: None
Return:
- Bool:
- true: Success
- false: Failure
PXR Set Foveation Parameter¶
Set Foveation parameter. It is suggested to use PXR Set Foveation Level interface predefined if not familiar with foveation rendering.
Blueprint:
Input:
- FVector2D:
- Foveation Gain Value: Gain of pixel density in X/Y axis. Higher gain set result in lower density of pixel (ranging in 0-100)
- Float:
- Foveation Area Value: Original resolution area centered in gazing point. Higher value indicates a larger area of original resolution
- Foveation Minimum Value: Minimum pixel density. Floor value of pixels in resolution downgrading area (ranging in 0-100)
Output: None
Return:
- Bool:
- true: Success
- false: Failure
7.6 Entitlement check relevant libary¶
Pico Entitlement Verify App Delegate¶
Return the result of entitlement verify result.
Blueprint:

Input:
- Delegate:
- On Verify App Callback: 绑Bind event of entitlement check as shown below:
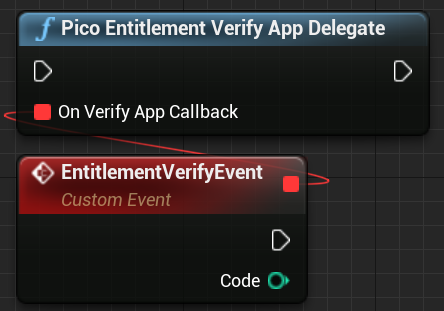
- Callback event params:
- Int: Varification result code:
- 0: Success
- -2: Service doesn’t exist
- -3: Service binding failure
- -4: Catch exception code
- -5: Time out
- 10: The package name is missing
- 11: The App ID is missing
- 13: The package name and App ID do not match
- 20: The user has not logged in
- 21: The user has not purchased
- 31: The app was not found
- 32: The purchase SN number does not match the device SN number
- Int: Varification result code:
Output: None
Return: None
7.7 SplashScreen relevant libary¶
The following APIs are available in PXRHMD category. Note: Before using SplashScreen APIs, refer to chapter 8.7 for eye tracking relevant configurations.
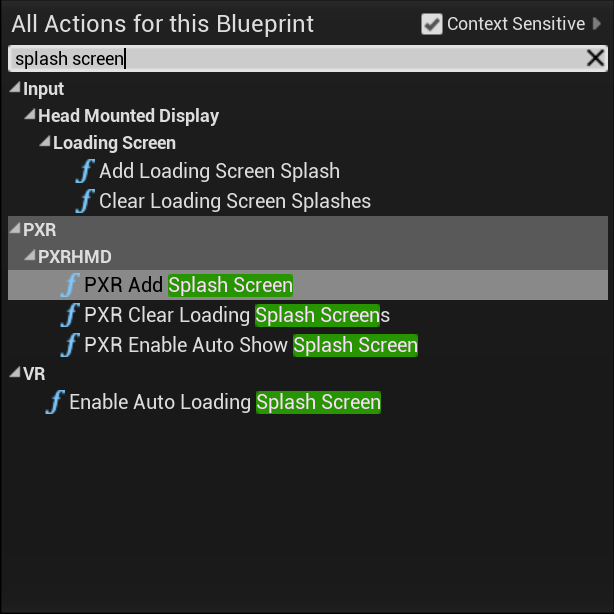
Figure 7.6 SplashScreen relevant libary
PXR Clear Loading Splash Screens¶
Clear current configuration for Splash Screen (Set to not display automatically)
Blueprint:
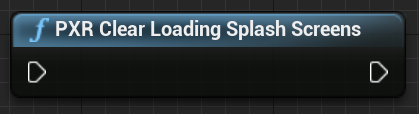
Input: None
Output: None
Return: None
PXR Enable Auto Show Splash Screen¶
Set whether to display Splash Screen automatically
Blueprint:
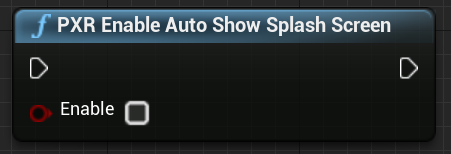
Input:
- Bool:
- Enable:
- Checked:true,Enable to display automatically
- Unchecked:false,Disable to display automatically
- Enable:
Output: None
Return: None
PXR Add Splash Screen¶
Add splash screen and set splash screen properties. Note: in Unreal 4.26, using this interface might lead to incorrect behaviors. It’s suggested to use Unreal standard API Add Loading Screen Splash to add Splash Screen in Unreal 4.26 and newer versions.
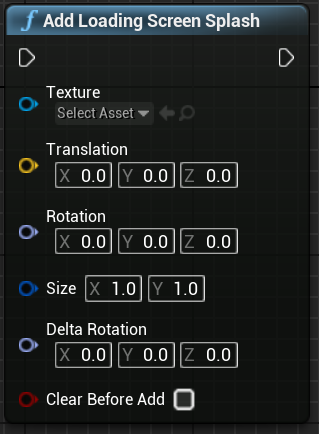
Blueprint:
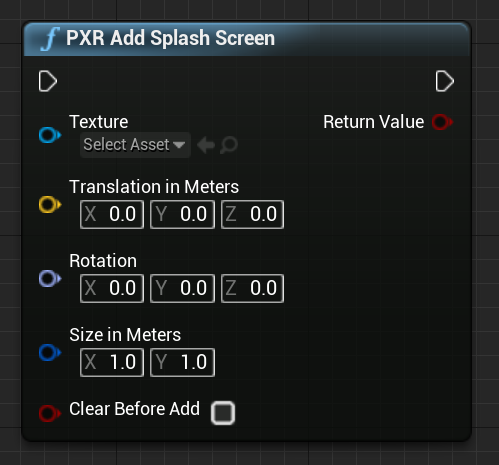
Input:
- Texture 2D Object reference:
- Texture: The texture that needs to be added to splash screen
- FVector:
- Translation in Meters: Offset from origin of center of Splash Screen (unit in meter)
- FRotator:
- Rotation: Initial Splash screen rotation. Origin is splash screen center
- FVector2D:
- Size in Meters: SplashScreen size in meters
- Bool:
- Clear Before Add: Whether to clear all splash images before adding a new splash screens
Output: None
Return:
- Bool:
- true: Success in adding splash screen
- false: Failure in adding splash screen
7.8 Boundary relevant libary¶
The following APIs are available in PXRHMD category.
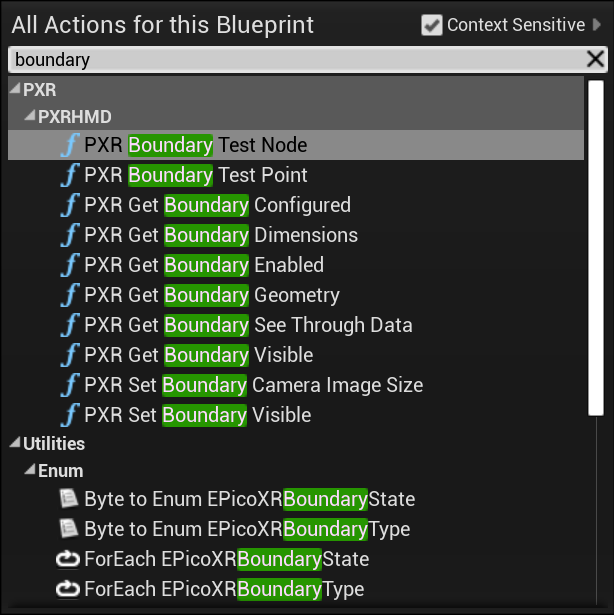
Figure 7.7 Boundary relevant libary
PXR Set Boundary Visible¶
Set result whether the safety border grid is resident
Blueprint:
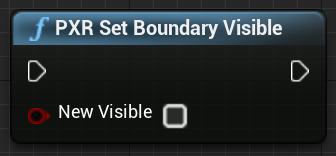
Input:
- Bool:
- New Visible:
- Checked:true,Safety boundary visible constantly enabled
- Unchecked:false,Safety boundary visible constantly disabled
- New Visible:
Output: None
Return: None
PXR Get Boundary Visible¶
Get result whether the safety border grid is resident
Blueprint:
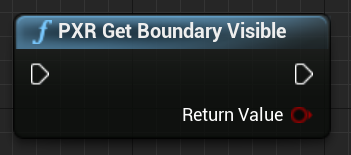
Input: None
Output: None
Return:
- Bool:
- true:Safety boundary visible constantly enabled
- false:Safety boundary visible constantly disabled
PXR Get Boundary Dimensions¶
Get the dimension of the customized Safety Boundary
Blueprint:
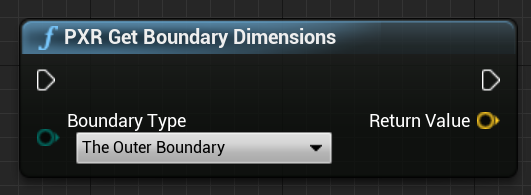
Input:
- Enum:
- Boundary Type(EPicoXRBoundaryType):
- The Outer Boundary:Safety boundary(i.e. Quick setting of safety boundary)
- The Play Area Boundary:Maximum internal rectangle of customized safety boundary(None if using quick setting safety boundary)
- Boundary Type(EPicoXRBoundaryType):
Output: None
Return:
- Vector:Describe rectangle by x and y value of FVecto,x:Long edge,y:1,z:Short edge,For situ safety boundary,Vector is(0,0,0)
PXR Get Boundary Through Data¶
Get the image of the left and right cameras (Not supported for Vulkan)
Blueprint:
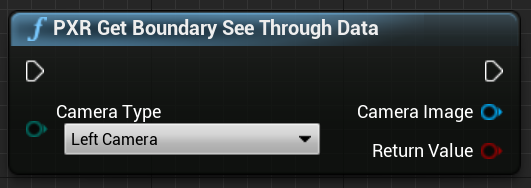
Input:
- Enum:
- Camera Type(EPicoXRCameraType):
- Left:Left camera
- Right:Right camera
- Camera Type(EPicoXRCameraType):
Output:
- Texture2D Object reference:
- Camera Image:Camera images acquired
Return:
- Bool:
- true:Success
- false:Failure
PXR Get Boundary Enabled¶
Get the result of whether safety boundary is on
Blueprint:
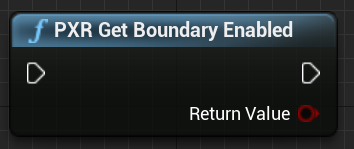
Input: None
Output: None
Return:
- Bool:
- true:Safety boundary enabled
- false:Safety boundary disabled
PXR Get Boundary Configured¶
Get whether the system has a valid security boundary
Blueprint:
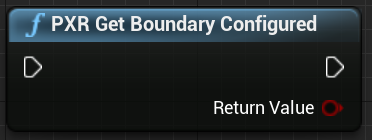
Input: None
Output: None
Return:
- Bool:
- true:Valid safety boundary persist
- false:No valid safety boundary persist
PXR Get Boundary Geometry¶
Get an array of safe zone coordinates
Blueprint:
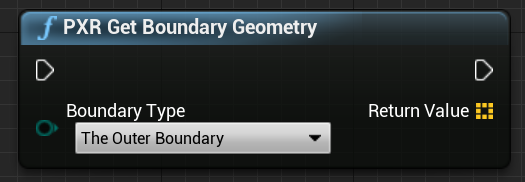
Input:
- Enum:
- Boundary Type(EPicoXRBoundaryType):
- The Outer Boundary:Safety boundary(i.e. Quick setting of safety boundary)
- The Play Area Boundary:Maximum internal rectangle of customized safety boundary(None if using quick setting safety boundary)
- Boundary Type(EPicoXRBoundaryType):
Output: None
Return:
- Vector array:
- TArray<FVector>:Safety boundary coordinates
PXR Boundary Test Node¶
Check is a tracking node collides with safety boundary (Left hand, Right hand, Head). Return to the state in which the tracking node triggers a secure boundary
Blueprint:
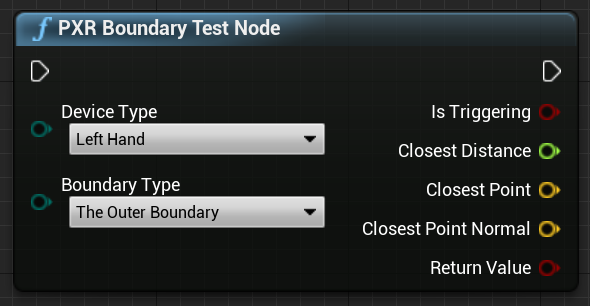
Input:
- Enum:
- Device Type(EPicoXRNodeType):
- LeftHand:Left hand
- RightHand:Right hand
- Head:Headset
- Device Type(EPicoXRNodeType):
- Enum:
- Boundary Type(EPicoXRBoundaryType):
- The Outer Boundary:Safety boundary(i.e. Quick setting of safety boundary)
- The Play Area Boundary:Maximum internal rectangle of customized safety boundary(None if using quick setting safety boundary)
- Boundary Type(EPicoXRBoundaryType):
Output:
- Bool :
- Is Triggering:Whether safety boundary is triggered
- Float:
- Closest Distance:Closest distance of tracked node to safety boundary
- FVector :
- Closest Point:Closest point of tracking node to specified safety boundary
- Closest Point Normal:Normal of closest point of tracking node to specified safety boundary
Return:
- Bool:
- true:Success
- false:Failure
PXR Boundary Test Point¶
Return to the state in which the tracking point triggers a secure boundary in Unreal coordinate system. This will out output intersect result if safety boundary is triggered.
Blueprint:
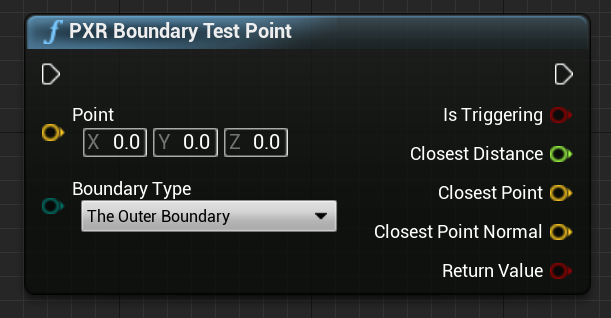
Input:
- FVector:
- Point:Tracking test point under Unreal coordinate
- Enum:
- Boundary Type(EPicoXRBoundaryType):
- The Outer Boundary:Safety boundary(i.e. Quick setting of safety boundary)
- The Play Area Boundary:Maximum internal rectangle of customized safety boundary(None if using quick setting safety boundary)
- Boundary Type(EPicoXRBoundaryType):
Output:
- Bool:
- Is Triggering: Whether safety boundary is triggered
- Float:
- Closest Distance: Closest distance of test node to safety boundary
- FVector:
- Closest Point:Closest point of test node to specified safety boundary
- Closest Point Normal:Normal of closest point of test node to specified safety boundary
Return:
- Bool:
- true:Success
- false:Failure
7.9 Advanced guardian relevant libary¶
The following APIs are available in PXRHMD category.
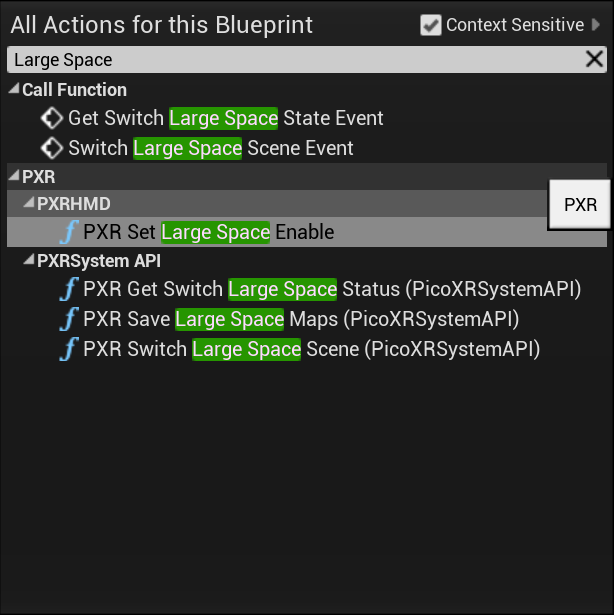
Figure 7.8 Large Space relevant libary
PXR Set Large Space Enable¶
Set whether current application supports Large Space
Blueprint:
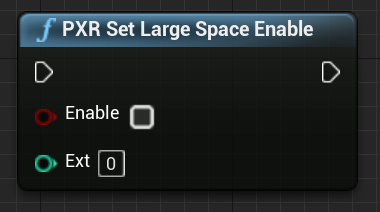
Input:
- Bool:
- Enable:
- Checked:true,Support large space
- Unchecked:false,Not support large space
- Enable:
- Int:
- Ext: Preserved for extensions
Output: None
Return: None
PXR Switch Large Space Scene¶
Enable/disable advanced guardian mode at system level. Component PicoXR System API is required. Bind a callback event to acquire switch result.
Blueprint:
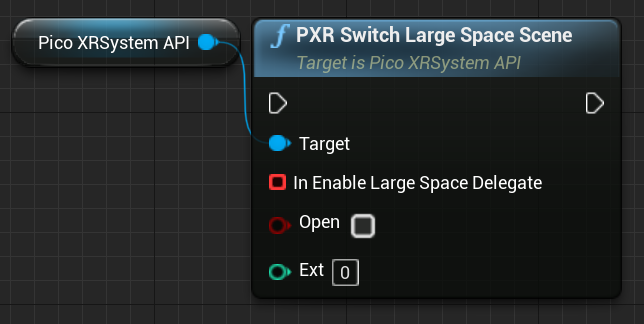
Input:
Delegate:
- In Enable Large Space Delegate: Bind event of switching large space as shown:
- Callback event params:
- Bool:
- true:Success
- false:Failure
- Bool:
Bool:
- Open:
- Checked:true,Enable large space
- Unchecked:false,Disable large space
- Open:
Int:
- Ext: Preserved for extensions
Output: None
Return: None
PXR Get Switch Large Space Status¶
Get the current advanced guardian status. “0” means disabled, “1” means enabled. Component PicoXR System API is required. Bind a callback event to acquire large space status.
Blueprint:
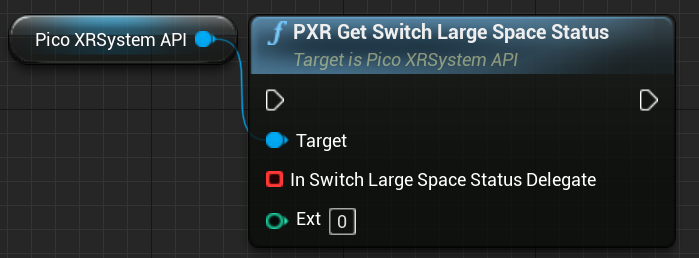
Input:
Delegate:
- In Enable Large Space Status Delegate: Bind event of acquiring large space status:
- Callback event params:
- FString:
- “0”: disabled
- “1”: enabled
- FString:
Int:
- Ext: Preserved for extensions
Output: None
Return: None
PXR Save Large Space Maps¶
Save Advanced Guardian maps. Component PicoXR System API is required.
Blueprint:
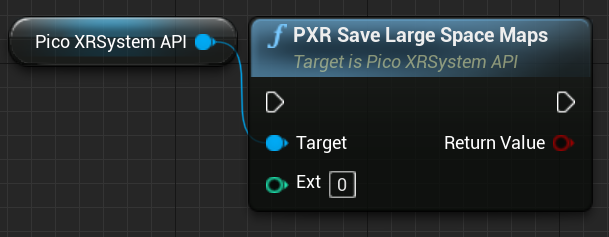
Input:
- Int:
- Ext: Preserved for extensions
Output: None
Return:
- Bool:
- true:Success
- false:Failure
PXR Export Maps¶
Export the map file, the exported file is stored in path: internal storage /maps/export Component PicoXR System API is required. Bind a callback event to acquire exported maps.
Blueprint:
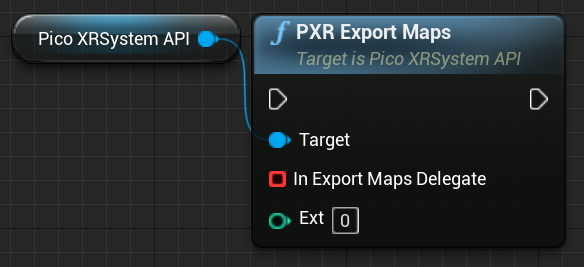
Input:
Delegate:
- In Export Maps Delegate: Bind exported map resul event:
- Callback event params:
- FString:
- true:Success
- false:Failure
- FString:
Int:
- Ext: Preserved for extensions
Output: None
Return: None
PXR Import Maps¶
To import a map file, the file should be copied to the path: internal storage/maps/ beforehand, and then execute the import method. Component PicoXR System API is required. Bind a callback event to acquire exported maps.
Blueprint:
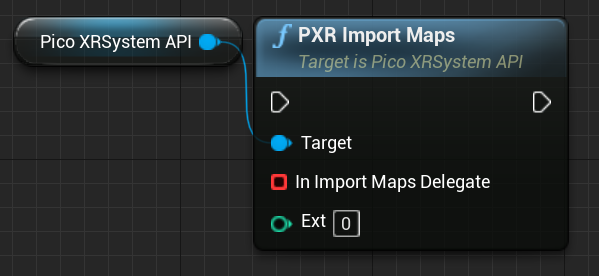
Input:
Delegate:
- In Import Maps Delegate: Bind import map callback event:
- Callback event params:
- FString:
- true:Success
- false:Failure
- FString:
Int:
- Ext: Preserved for extensions
Output: None
Return: None
PXR Get Predicted Main Sensor State¶
Get the posture data of the device in a fixed coordinate system
Blueprint:
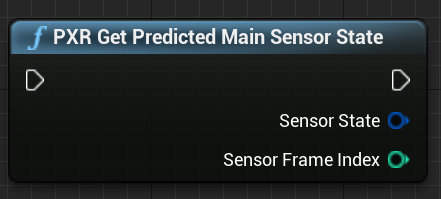
Input: None
Output:
Struct:
SensorState: FPxrSensorState
- Int: status:
- 0:pose/globalPose data not available
- 1: only pose data available
- 3:pose/globalPose available
- FQuat:poseQuat:Headset pose
- FVector:poseVector:Headset position vector
- FQuat:globalPoseQuat:Relative pose of headset to boundary
- FVector:globalPoseVector:Relative position of headset to boundary
- FVector:angularVelocity:Angular velocity
- FVector:linearVelocity:Linear velocity
- FVector:angularAcceleration:Angular acceleration
- FVector:linearAcceleration:Linear acceleration
- Int:poseTimeStampNs: Timestamps acquired from about params (ns)
Int:
- Sensor Frame Index: Index of sensor data of current frame.
Return: None
7.10.1 Common interface¶
PXR Get Device Info¶
Get info of currently using device.
Blueprint:
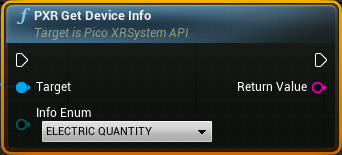
Input:
Device info type is shown in the following table:
ESystemInfoEnum | Implication |
---|---|
ELECTRIC_QUANTITY | Battery level |
PUI_VERSION | PUI version |
EQUIPMENT_MODEL | Device model |
EQUIPMENT_SN | Device SN |
CUSTOMER_SN | Customer SN |
INTERNAL_STORAGE_SPACE_OF_THE_DEVICE | Storage space of the device |
DEVICE_BLUETOOTH_STATUS | Bluetooth status |
BLUETOOTH_NAME_CONNECTED | Name of bluetooth connection |
BLUETOOTH_MAC_ADDRESS | Mac address of bluetooth |
DEVICE_WIFI_STATUS | WIFI connection status |
WIFI_NAME_CONNECTED | Name of connected WiFi |
WLAN_MAC_ADDRESS | Mac address of WLAN |
DEVICE_IP | Device IP |
SystemInfoEnum.CHARGING_STATUS | Charging status charged-2,uncharged-3 |
Output: None
Return:
- FString: Return device info string based on given ESystemInfoEnum enum. e.g. Using EQUIPMENT_SN returns SN code
PXR Set Auto Connect Wifi¶
Connect to a specified Wi-Fi. Bind delegate event to get the setup result.
Blueprint:
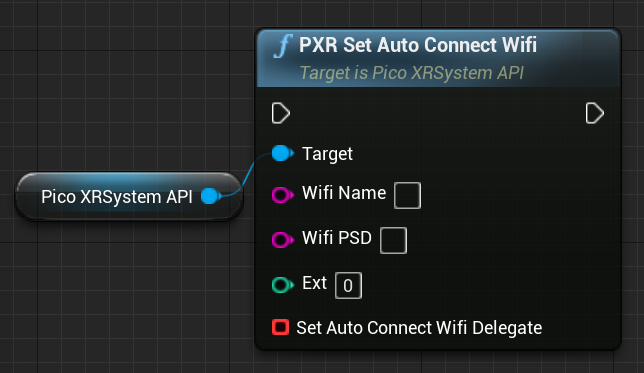
Input:
FString:
- Wifi Name: ID of specified WiFi
- Wifi PSD: password of specified WiFi
Int:
- Ext: Preserved for extensions
Delegate:
- Set Auto Connect Wifi Delegate: Event for auto connecting WiFi
Callback event params:
- Bool:
- True: Success
- False: Failure
- Bool:
Output: None
Return: None
PXR Clear Auto Connect Wifi¶
Disable auto- connect to a specified WiFi
Blueprint:
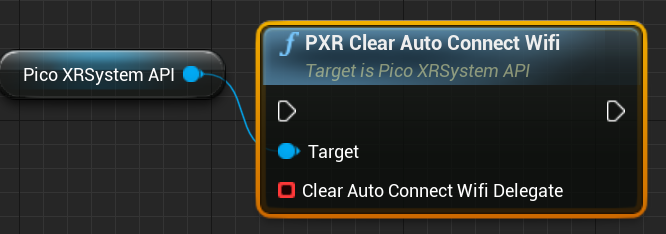
Input:
Delegate:
- Clear Auto Connect Wifi Delegate: clear setup for auto-connect to specified Wifi as shown below:
Callback event params:
- Bool:
- True: Success
- False: Failure
- Bool:
Output: None
Return: None
PXR Set Home Key¶
Set Home key customized function. It will redefine Home key and affect the function of Home key defined by the system. Please use this function with discretion. Set EventEnum for single click and double click correspondingly. Set FunctionEnum to configure the expected function. Bind the callback to get set result.
Blueprint:
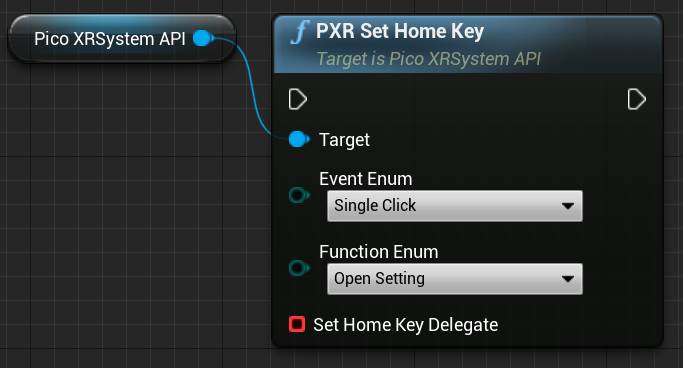
Input:
Enum:
- EventEnum( EHomeEventEnum):
- HOME_SINGLE_CLICK (“Single Click”): Single click on Home button
- HOME_DOUBLE_CLICK (“Double Click”) : Single click on Home button
- FunctionEnum(EHomeFunctionEnum):
- VALUE_HOME_GO_TO_SETTING (“Open Setting”):Open setting
- VALUE_HOME_RECENTER ( “Recenter”):Recenter
- VALUE_HOME_DISABLE (“Disable”):Disable Home key
- VALUE_HOME_GO_TO_HOME (“Open Launcher”):Open launcher
- EventEnum( EHomeEventEnum):
Delegate:
- Set Home Key Delegate: Bind delegate event for Home Key configuration as shown below:
Callback event params:
- Bool:
- True: Success
- False: Failure
- Bool:
Output: None
Return: None
PXR Home Key All¶
Set Home key customized function. It will redefine Home key and affect the function of Home key defined by the system. Please use this function with discretion. Set EventEnum for single click and double click correspondingly. Set TimeSetup to configure the interval of double click. Set FunctionEnum to configure the expected function. Bind the callback to get set result.
Blueprint:
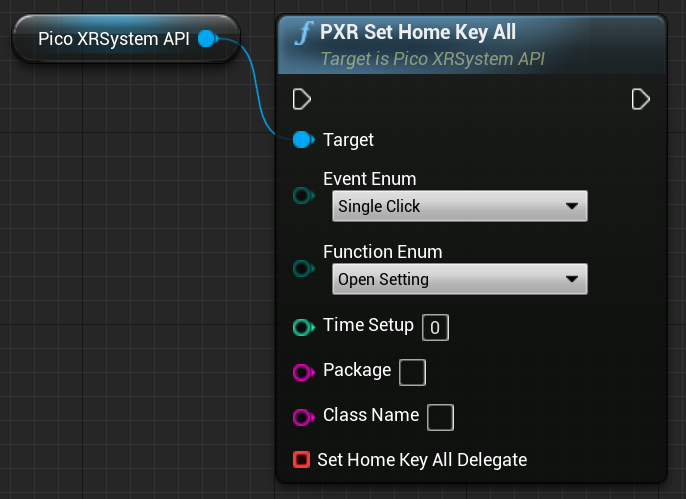
Input:
Enum:
- EventEnum( EHomeEventEnum):
- HOME_SINGLE_CLICK (“Single Click”): Single click on Home button
- HOME_DOUBLE_CLICK (“Double Click”) : Single click on Home button
- FunctionEnum(EHomeFunctionEnum):
- VALUE_HOME_GO_TO_SETTING (“Open Setting”):Open setting
- VALUE_HOME_RECENTER ( “Recenter”):Recenter
- VALUE_HOME_DISABLE (“Disable”):Disable Home key
- VALUE_HOME_GO_TO_HOME (“Open Launcher”):Open launcher
- EventEnum( EHomeEventEnum):
Int:
- TimeSetup only double click and long pressing (long pressing has been deprecated) requires time setup, use 0 if it’s single click
FString:
- Package: When function is set as “open application”, input specified package name (deprecaed).
- ClassName: When function is set as “open application”, input specified class name (deprecaed).
Delegate:
- SetHomeKeyAllDelegate: Bind event of calling SetHomeKeyAll:
Callback event params:
- Bool:
- True: Success
- False: Failure
- Bool:
Output: None
Return: None
PXR Disable Power Key¶
Set Power Key function. Bind delegate event to return the set result
Blueprint:
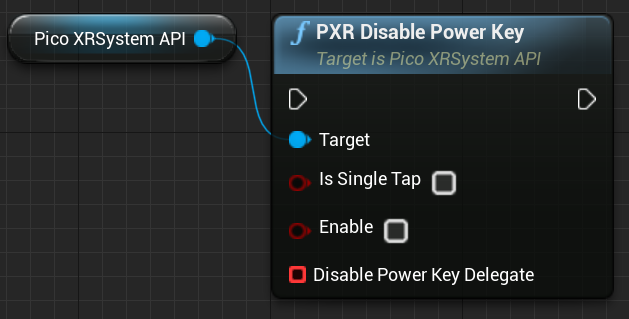
Input:
Bool:
- isSingleTap: Whether to set single-tap
- Checked: true: Single-tap to trigger
- Unchecked: false: Double-tap to trigger
- Enable: Whether power key is enabled
- Checked: true: Enable PowerKey
- Unchecked: false: Disable PowerKey
- isSingleTap: Whether to set single-tap
Delegate:
- Disable Power Key Delegate: Bind delegate event for calling DisablePower blueprint:
- Callback event params:
- Int:
- 0: Success
- 1: Failure
- Int:
Output: None
Return: None
PXR Set Screen Off Delay¶
Set duration of screen off delay
Blueprint:
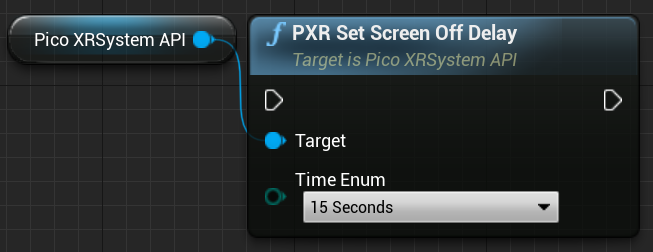
Input:
- Enum:
- TimeEnum(ESleepDelayTimeEnum):
- FIFTEEN:15 seconds
- THIRTY:30 seconds
- SIXTY:1 minute
- THREE_HUNDRED:5 minute
- SIX_HUNDRED:10 minute
- ONE_THOUSAND_AND_EIGHT_HUNDRED:30 minute
- NEVER:Never turn off screen
- TimeEnum(ESleepDelayTimeEnum):
Output: None
Return: None
PXR Set Sleep Delay¶
Set duration of sleep off delay
Blueprint:
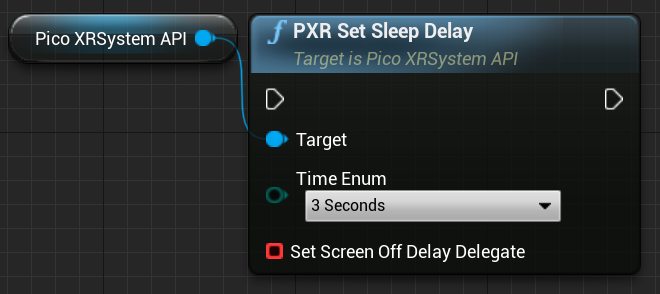
Input:
Enum:
- TimeEnum(ESleepDelayTimeEnum):
- FIFTEEN:15 seconds
- THIRTY:30 seconds
- SIXTY:1 minute
- THREE_HUNDRED:5 minute
- SIX_HUNDRED:10 minute
- ONE_THOUSAND_AND_EIGHT_HUNDRED:30 minute
- NEVER:Never sleep
- TimeEnum(ESleepDelayTimeEnum):
Delegate:
- SetScreenOffDelayDelegate: Set delegate for sleep delay setup as shown below:
Callback event params:
- Int:
- 0: Success
- 1: Failure
- 10: Time to set is too long
- Int:
Output: None
Return: None
PXR Switch System Function¶
Switch commonly used system options
Blueprint:
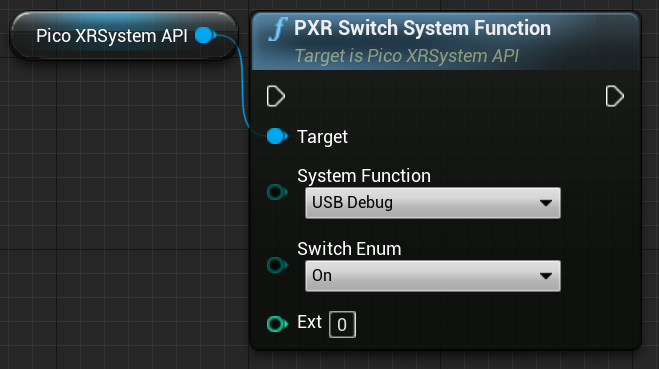
Input:
- Enum:
- SystemFunction(ESystemFunctionSwitchEnum):
- SFS_USB (“USB Debug”):USB debugging switch
- SFS_AUTOSLEEP (“Auto Sleep”):Auto sleep switch
- SFS_SCREENON_CHARGING (“ScreenOn Charging”):Screenon charging switch
- SFS_OTG_CHARGING (“OTG Charging”):OTG charging switch
- SFS_RETURN_MENU_IN_2DMODE (“Show Back Menu in 2D mode”):Show Back Menu in 2D mode switch
- SFS_COMBINATION_KEY (“Combination Key”):Combination key switch
- SFS_CALIBRATION_WITH_POWER_ON (“Calibration wiht power on”):Calibration with power on switch
- SFS_SYSTEM_UPDATE (“System Update”):System update switch
- SFS_CAST_SERVICE (“Cast Service”):Cast service switch. This property does not take effect when the industry solution switch is on.Cast service switch. This property does not take effect when the industry solution switch is on.
- SFS_EYE_PROTECTION (“Eye Protection”):Eye protection switch
- SFS_SECURITY_ZONE_PERMANENTLY (“Security Zone Permanently”):Security zone permanently on/ off switch
- SFS_Auto_Calibration (“Auto Calibration”):Auto calibration switch
- SFS_USB_BOOT (“USB Boot”):USB Boot switch
- SwitchEnum(ESwitchEnum):
- S_ON(”On”):Switch on
- S_OFF (“Off”) :Switch off
- SystemFunction(ESystemFunctionSwitchEnum):
- Int:
- Ext: Reserved for extensions
Output: None
Return: None
PXR Set Usb Configuration Option¶
Set USB configuration (MTP, charging). MTP mode allows transferring media files. Changing mode doesn’t allow file transfer.
Blueprint:
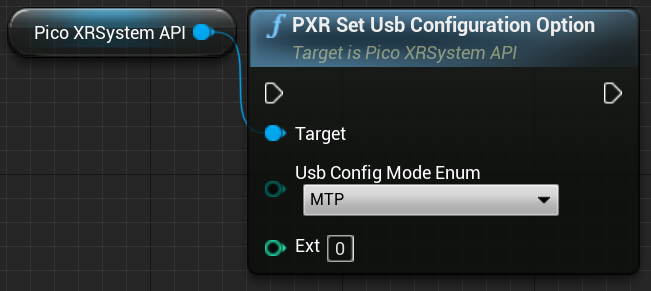
Input:
- Enum:
- Usb Config Mode Enum(EUSBConfigModeEnum):
- MTP:Media Transfer Protocol mode
- CHARGE:Charging mode
- Int:
- Ext: Preserved for extensions
- Usb Config Mode Enum(EUSBConfigModeEnum):
Output: None
Return: None
PXR Write Config File to Data Local¶
Write configuration file to /data/local/tmp path. Bind delegate event to get set result. Note: only enterprise devices can use
Blueprint:
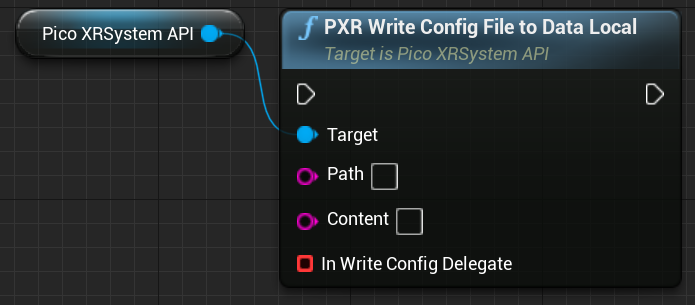
Input:
FString:
- Path:Path that the configuration file save
- Content:Contents to write into the configuration file
Delegate:
- In Write Config Delegate:bind delegate event of writing configuration file
- Callback event params:
- Bool:
- true:Success
- false:Failure
- Bool:
Output: None
Return: None
PXR Reset All Key to Default¶
Restore system key configurations. Bind a delegate event to get reset result
Blueprint:
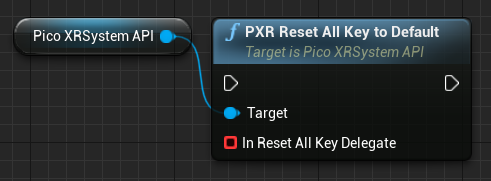
Input:
Delegate:
- In Reset All Key Delegate: Bind event to check reset all keys event
Callback event params:
- Bool:
- True: Success
- False: Failure
- Bool:
Output: None
Return: None
PXR Enable Enter Key¶
Cancel disabling enter key on headset. Call this interface to re-enable headset button if disabled before.
Blueprint:
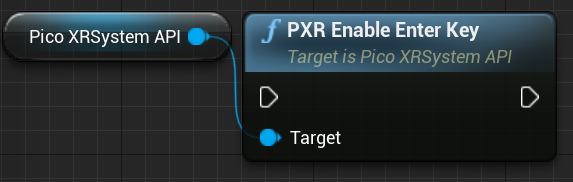
Input: None
Output: None
Return: None
PXR Disable Enter Key¶
Disable enter key on headset.
Blueprint:
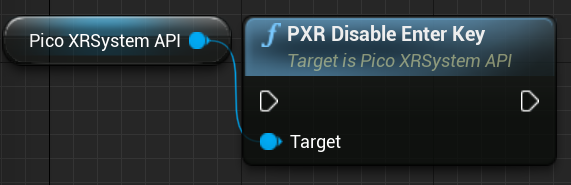
Input: None
Output: None
Return: None
PXR Disable Volume Key¶
Disable volume key on headset.
Blueprint:
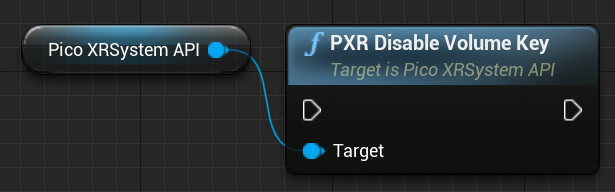
Input: None
Output: None
Return: None
PXR Enable Volume Key¶
Cancel disabling enter key on headset. Call this interface to re-enable headset button if disabled before.
Blueprint:
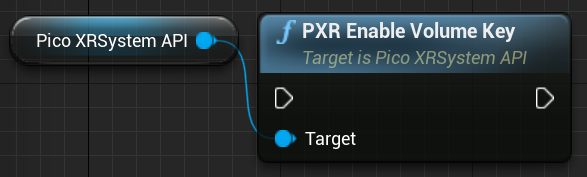
Input: None
Output: None
Return: None
PXR Enable Back Key¶
Cancel disabling back key on headset. Call this interface to re-enable headset button if disabled before.
Blueprint:
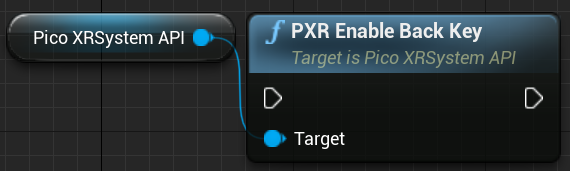
Input: None
Output: None
Return: None
PXR Get Device SN¶
Get device SN code
Blueprint:
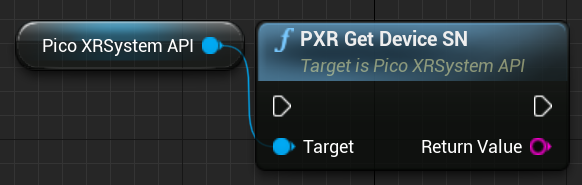
Input: None
Output: None
Return:
- FString:
- SN code string
PXR Get Current Brightness¶
Get current brightness of screen
Blueprint:
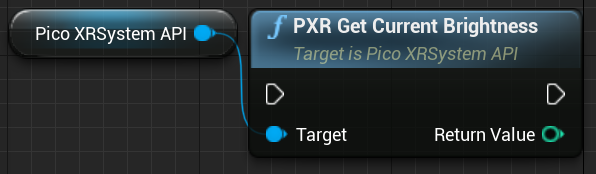
Input: None
Output: None
Return:
- Int:
- Current brightness(Range:0-255)
PXR Set Brightness¶
Set the current brightness of screen. Note: first time to call this interface requires system permission
Blueprint:
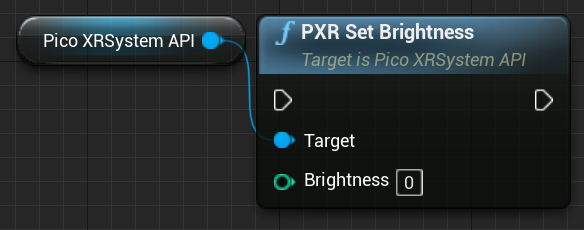
Input:
- Int:
- Brightness: brightness(Range:0-255)
Output: None
Return: None
PXR Get Current Volume¶
Get current volume
Blueprint:
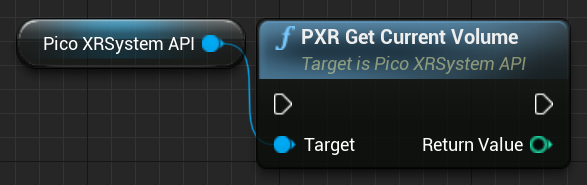
Input: None
Output:
- Int:
- Current volume (range: 1-15)
Return: None
PXR Get Max Volume¶
Get maximum volume of the headset
Blueprint:
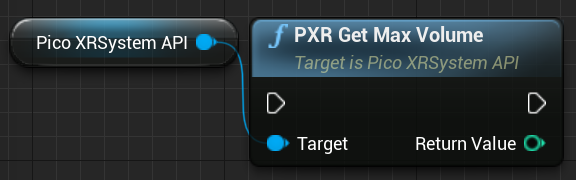
Input: None
Output: None
Return:
- Int:
- Maximum volume
PXR Set Volume¶
Set volume of the headset
Blueprint:
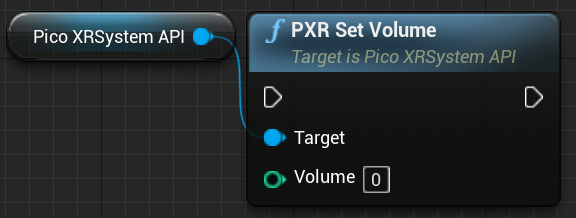
Input:
- Int:
- Volume: volume value (range 1-15)
Output: None
Return: None
PXR Kill Background Apps with White List¶
Kill background application excluding white listed apps.
Blueprint:
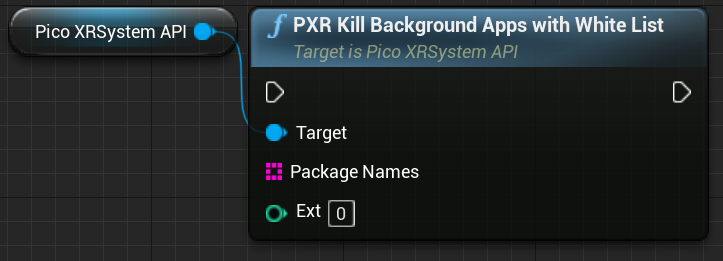
Input:
- FString Array(TArray<FString>):
- PackageNames:Array of package names in white list
- Int:
- Ext:Preserved for extension
Output: None
Return: None
7.10.2 Protected interface¶
Notes:The following interfaces are protected interfaces. Before calling them, it is required to check the “Use Pico Advance Interface” option in Project Settings→Plugin→PicoXR Settings. If this option is checked, your app will not be available on the Pico Store. If your app needs to be distributed through the Pico Store, please do not check the “Use Pico Advance Interface” option or use these Interfaces.
PXR Set Device Action¶
Reboot or power off device. Bind delegate event to get result.
Blueprint:
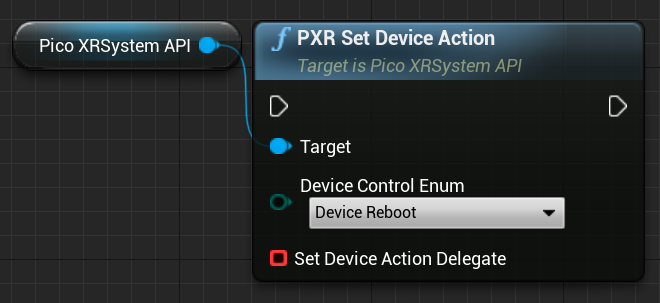
Input:
Enum:
- Device Control Enum(EDeviceControlEnum):
- DEVICE_CONTROL_REBOOT (“Device Reboot”):Reboot device
- DEVICE_CONTROL_SHUTDOWN (“Device ShutDown”):Power off device
- Device Control Enum(EDeviceControlEnum):
Delegate:
- Set Device Action Delegate:Bind device action callback event:
- Callback event params:
- int:
- 0:Success
- 1:Failure
- int:
Output: None
Return: None
PXR App Manager¶
Silent install or uninstall application. Bind delegate event to get installing result.
Blueprint:
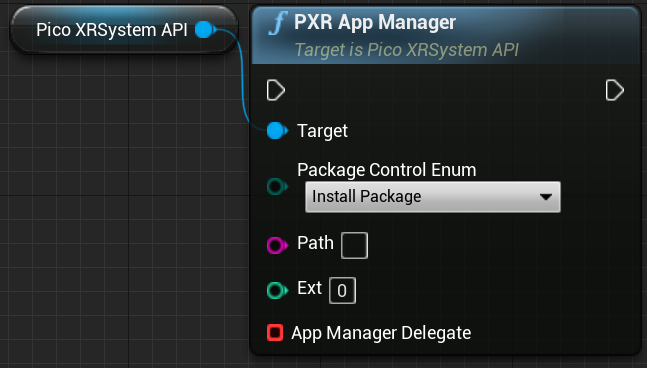
Input:
Enum:
- Package Control Enum(EPackageControlEnum):
- PACKAGE_SILENCE_INSTALL (“Install Package”):Silent install apk file
- PACKAGE_SILENCE_UNINSTALL (“Uninstall Package”):Silent uninstall apk file
- Package Control Enum(EPackageControlEnum):
FString:
- Path:Path of apk file to install or package name to uninstall
Int:
- Ext:Preserved for extensions
delegate
- App Manager Delegate:Bind silent install/uninstal callback event
- Callback event params:
- Int:
- 0:Success
- 1:Failure
- Int:
Output: None
Return: None
PXR Screen Off¶
Switch screen off. Note: First-time calling will prompt power management permission check.
Blueprint:
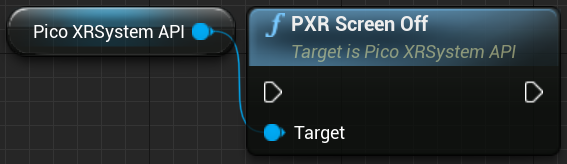
Input: None
Output: None
Return: None